Bucles y bucles de salida en VBA
-
Bucle
For
de VBA -
Bucle VBA
Do Until
-
Bucle
Do While
de VBA -
Utilizando el comando
Exit
para forzar la detención del bucle en VBA
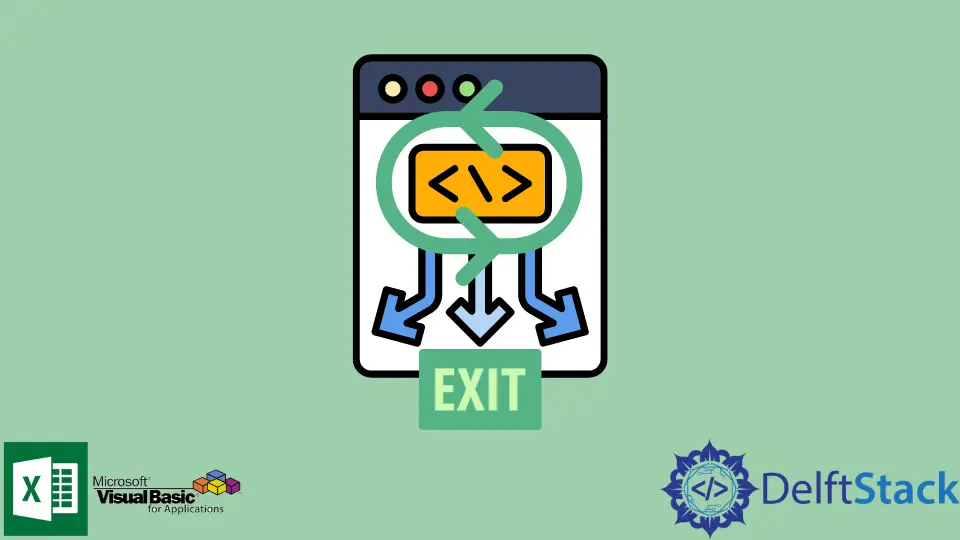
Los bucles en la programación de computadoras son muy importantes. Permiten ejecutar tareas repetitivas con un mínimo de líneas de código. La necesidad de loops en las computadoras surge por varias razones dependiendo de las tareas a realizar.
A pesar de que son tan poderosos, son simples en contexto y sintaxis.
En VBA, hay tres bucles que se pueden utilizar.
- Bucle
For
- Bucle dentro de límites predefinidos o fijos - Bucle
Do While
: realiza un bucle mientras la condición esTrue
. - Bucle
Do Until
: bucles hasta que la condición seaTrue
.
Bucle For
de VBA
Sintaxis:
For [ counter ] = [start] To [end] Step [ step ]
[ statements ]
[ Exit For ]
Next [ counter ]
Parámetros:
[ counter ] |
Requerido. Por lo general, una variable entera o larga que contiene el valor actual del contador |
[ start ] |
Requerido. El valor inicial del contador. |
[end] |
Requerido. El valor final del contador. |
[ statements ] |
El bloque de código para ejecutar dentro del bucle |
[ Exit For ] |
Opcional. Forzar el bucle para detenerse y salir del bloque de código de bucle |
[ step ] |
Opcional. El incremento del contador en cada iteración. Si no se declara, el valor es 1 . |
El ejemplo del bucle For
:
Este bloque de código aceptará un valor entero como su número inicial, luego disminuirá el número hasta cero usando un bucle For
. Imprimirá el resultado de cada iteración.
Sub ReduceToZero(numStart As Integer)
Dim i As Integer
For i = numStart To 0 Step -1
Debug.Print "The number is now " & i
If i = 0 Then
Debug.Print ("Done!")
End If
Next i
End Sub
Sub testSub()
Call ReduceToZero(10)
End Sub
Producción :
The number is now 10
The number is now 9
The number is now 8
The number is now 7
The number is now 6
The number is now 5
The number is now 4
The number is now 3
The number is now 2
The number is now 1
The number is now 0
Done!
Bucle VBA Do Until
Sintaxis:
Do Until [condition]
[statements]
[Exit Do]
Loop
Parámetros:
[condition] |
Requerido. La condición de que, una vez verdadera, saldrá del bloque de código. |
[statements] |
Requerido. El bloque de código a ejecutar. |
[Exit Do] |
Opcional. Forzar el bucle para que se detenga y salga del bloque de código |
Ejemplo de bucle Do Until
:
Este bloque de código aceptará un valor entero como su número inicial, luego aumentará el número hasta diez usando un bucle Do Until
. Imprimirá el resultado de cada iteración.
Sub IncrementToTen(numStart As Integer)
Dim i As Integer
i = numStart
Do Until i = 10 + 1
Debug.Print "The number is now " & i
i = i + 1
Loop
End Sub
Sub testSub()
Call IncrementToTen(-5)
End Sub
Salida testSub
:
The number is now -5
The number is now -4
The number is now -3
The number is now -2
The number is now -1
The number is now 0
The number is now 1
The number is now 2
The number is now 3
The number is now 4
The number is now 5
The number is now 6
The number is now 7
The number is now 8
The number is now 9
The number is now 10
Done!
Bucle Do While
de VBA
Sintaxis:
Do while [condition]
[statements]
[Exit Do]
Loop
Parámetros:
[condition] |
Requerido. La condición que debe ser verdadera para ejecutar el bloque de código. |
[statements] |
Requerido. El bloque de código a ejecutar. |
[Exit Do] |
Opcional. Forzar el bucle para que se detenga y salga del bloque de código |
El ejemplo del bucle Do While
:
Este bloque de código aceptará un valor entero como su número inicial, luego aumentará el número hasta diez usando un bucle Do While
. Imprimirá el resultado de cada iteración.
Sub IncrementToTen(numStart As Integer)
Dim i As Integer
i = numStart
Do While i < 10 + 1
Debug.Print "The number is now " & i
i = i + 1
Loop
Debug.Print "Done!"
End Sub
Sub testSub()
Call IncrementToTen(-9)
End Sub
Salida testSub
:
The number is now -9
The number is now -8
The number is now -7
The number is now -6
The number is now -5
The number is now -4
The number is now -3
The number is now -2
The number is now -1
The number is now 0
The number is now 1
The number is now 2
The number is now 3
The number is now 4
The number is now 5
The number is now 6
The number is now 7
The number is now 8
The number is now 9
The number is now 10
Done!
Utilizando el comando Exit
para forzar la detención del bucle en VBA
Cuando se trata de bucles, están sujetos a condiciones que el bucle seguirá ejecutando cuando se cumpla. Sin embargo, un escenario común es que necesitamos salir del bucle, incluso si las condiciones aún se cumplen. Una aplicación típica para esto es cuando se trata de métodos de Error-handling
, la técnica Stop-when-search
. Se necesita una salida inmediata del bucle para evitar más errores o ahorrar tiempo de ejecución.
A continuación, los bloques de código demostrarán el uso del comando Exit
para forzar la parada del bucle en un bucle Do
.
Este bloque de código tiene como objetivo detener el bucle cuando el cociente de dos números es uno. Estos dos números se generan aleatoriamente entre los valores enteros upperbound
y lowerbound
, los parámetros de la subrutina. Si el divisor generado (divis
) es cero, entonces se produciría un error ya que no se permite la división por cero. Para evitar que ocurra, usaremos el comando Exit
para terminar el bucle.
Sub StopWhenQuotientIsOne(upperbound As Integer, lowerbound As Integer)
Dim divid As Integer
Dim divis As Integer
Dim isOne As Boolean
divid = Int((upperbound - lowerbound + 1) * Rnd + lowerbound)
divis = Int((upperbound - lowerbound + 1) * Rnd + lowerbound)
Do Until isOne = True
If divis = 0 Then
Debug.Print "Illegal divisor. Exiting the Loop"
Exit Do
End If
Debug.Print divid / divis
If divid / divis = 1 Then
isOne = True
Debug.Print "Done! One is achieved."
End If
divid = Int((upperbound - lowerbound + 1) * Rnd + lowerbound)
divis = Int((upperbound - lowerbound + 1) * Rnd + lowerbound)
Loop
End Sub
Sub testSub()
Call StopWhenQuotientIsOne(4, -4)
End Sub
Salida testSub
(primera prueba):
-4
-0
-0.666666666666667
Illegal divisor. Exiting the Loop
Salida testSub
(4ª prueba):
-2
0
-3
1
Done! One is achieved.
El siguiente bloque de código demostrará el uso del comando Exit
para forzar la parada del bucle en un bucle For
.
En este ejemplo, la subrutina StopWhenNegative
requiere dos parámetros. Primero está el startNum
y luego EndNum
. El bucle iterará desde startNum
hasta EndNum
. Si el contador se vuelve negativo, el bucle se terminará con el comando Salir
.
Sub StopWhenNegative(startNum As Integer, EndNum As Integer)
Dim i As Integer
For i = startNum To EndNum Step -1
If i < 0 Then
Debug.Print "Opps, negative values detected. Exiting loop."
Exit For
End If
Debug.Print "The current number is :" & i
Next i
End Sub
Sub testSub()
Call StopWhenNegative(10, -5)
End Sub
Salida testSub
:
The current number is :10
The current number is :9
The current number is :8
The current number is :7
The current number is :6
The current number is :5
The current number is :4
The current number is :3
The current number is :2
The current number is :1
The current number is :0
Opps, negative values detected. Exiting loop.