Rango en Rust
-
Use la notación de rango
a..b
en Rust -
Use la notación de rango
a..=b
en Rust -
a..b
como buclefor
de C en Rust
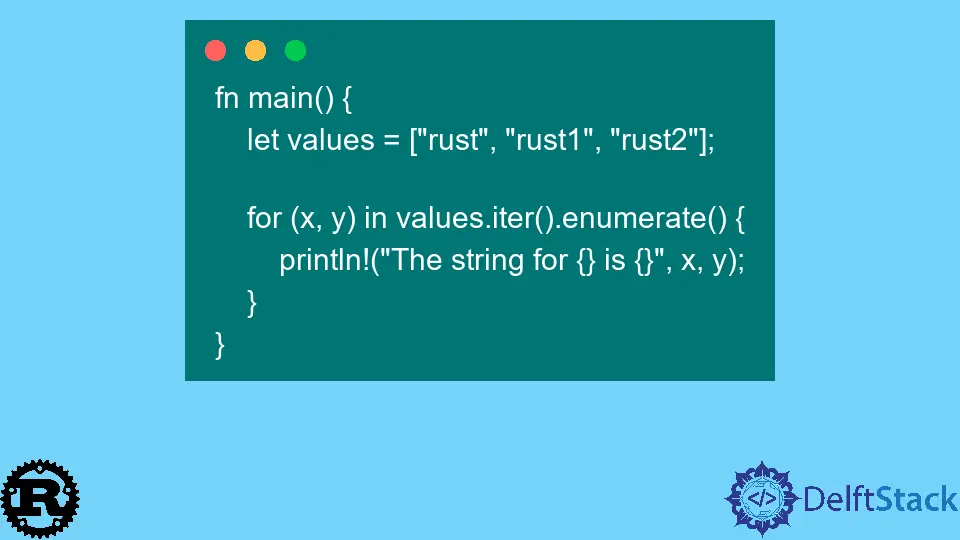
En este artículo, aprenderemos a crear un rango en Rust.
Use la notación de rango a..b
en Rust
Es posible iterar a través de un Iterador
utilizando la construcción for in
. Uno de los métodos más simples para generar un iterador es usar la notación de rango a..b
.
Esta es una de las notaciones más comunes. Esto produce valores que van desde a
(inclusivo) a b
(exclusivo) en incrementos de un paso.
La gama abarca tanto el principio como el final del tiempo. Y, si queremos que el rango incluya el final, podemos usar la sintaxis de rango a..=b
para hacer esto.
Ejemplo:
fn main() {
for i in 1..10 {
if i % 5 == 0 {
println!("hellothere");
} else if i % 2 == 0 {
println!("hello");
} else if i % 4 == 0 {
println!("there");
} else {
println!("{}", i);
}
}
}
Producción :
1
hello
3
hello
hellothere
hello
7
hello
9
Aquí, n
tomará los valores: 1, 2, ..., 10
en cada iteración.
Use la notación de rango a..=b
en Rust
En cambio, a..=b
también se puede usar para un rango
inclusive en ambos extremos. El código anterior también se puede escribir de la siguiente manera.
fn main() {
for i in 1..=10 {
if i % 5 == 0 {
println!("hellothere");
} else if i % 2 == 0 {
println!("hello");
} else if i % 4 == 0 {
println!("there");
} else {
println!("{}", i);
}
}
}
Producción :
1
hello
3
hello
hellothere
hello
7
hello
9
hellothere
Aquí, n
también tomará los valores: 1, 2, ..., 10
en cada iteración.
a..b
como bucle for
de C en Rust
La sintaxis a..b
se puede usar para iterar a través de un rango de números, similar al trabajo de los bucles for
de C.
for i in 0..3 {
println!("{}", i);
}
Si requerimos tanto el índice como el elemento de una matriz, el método Iterator::enumerate
es la forma idiomática de lograrlo.
fn main() {
let values = ["rust", "rust1", "rust2"];
for (x, y) in values.iter().enumerate() {
println!("The string for {} is {}", x, y);
}
}
Producción :
The string for 0 is rust
The string for 1 is rust1
The string for 2 is rust2