Leer un archivo de texto línea por línea en R
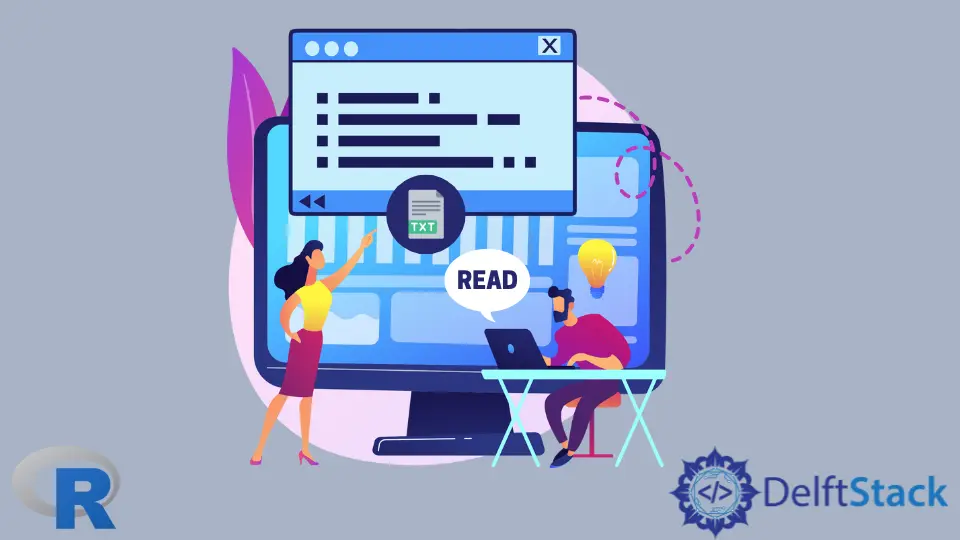
En la programación de R, leer archivos de texto línea por línea es una operación común en tareas de análisis y manipulación de datos. Este artículo discutirá el método comúnmente utilizado para leer un archivo de texto línea por línea en R.
Usar la función readLines()
para leer un archivo de texto línea por línea en R
Utilizaremos la función readLines()
mientras se lean las líneas. Como no conocemos el número de líneas, necesitamos saber cuándo se llega al final del archivo.
La función readLines()
devuelve un vector de caracteres vacío character(0)
cuando no hay más líneas en el archivo.
En este punto, saldremos del bucle con un break
. Tenga en cuenta que el bucle no se interrumpirá en líneas en blanco, ya que estas tienen los marcadores de fin de línea.
Utilizaremos la función identical()
en cada iteración del bucle para verificar si la línea es idéntica a un character(0)
.
Si lo es, break
; de lo contrario, procesamos la línea. En el ejemplo, la imprimimos.
Según la documentación, la última línea del archivo de texto debe tener un marcador de fin de línea para que la función readLines()
funcione de manera consistente.
Primero, cree un archivo de texto de muestra con el siguiente texto y guárdelo con codificación UTF-8 y un nombre de archivo adecuado (necesitaremos reemplazar el nombre del archivo en el código correspondientemente).
The first line.
The second line.
The third line.
Last line.
En el código de ejemplo, haremos lo siguiente.
-
Crear una conexión y abrirla.
-
Recorrer el archivo, una línea a la vez, imprimiéndola.
-
Salir del bucle cuando la línea sea un
character(0)
. -
Cerrar la conexión. Una conexión abierta explícitamente debe ser cerrada explícitamente.
Código de ejemplo:
# Explicitly create and open a connection.
# REPLACE THE FILE NAME AS PER YOUR FILE.
myCon = file(description = "filename.txt", open="r", blocking = TRUE)
# The position in the connection advances to the next line on each iteration.
# Loop till the line is the empty vector, character(0).
repeat{
pl = readLines(myCon, n = 1) # Read one line from the connection.
if(identical(pl, character(0))){break} # If the line is empty, exit.
print(pl) # Otherwise, print and repeat next iteration.
}
# Explicitly opened connection needs to be explicitly closed.
close(myCon)
rm(myCon) # Removes the connection object from memory.
Salida:
The first line.
The second line.
The third line.
Last line.
Tengamos otro ejemplo.
Código de ejemplo:
# Specify the path to your text file
file_path <- "your_file.txt"
# Use the readLines function to read the file line by line
# It reads the entire content of the specified file and stores each line as an element in a character vector named lines
lines <- readLines(file_path)
# Loop through the lines and process them
for (line in lines) {
# Process each line here (e.g., print it)
cat(line, "\n")
}
Cuando ejecutes este código, leerá el archivo de texto línea por línea y mostrará cada línea.
Resultado:
The first line.
The second line.
The third line.
Last line.
Recuerda reemplazar your_file.txt
con la ruta de archivo real del archivo de texto de muestra con el que deseas trabajar.