Usar el operador %*% en R
- Matrix y sus dimensiones en R
-
Usar el Operador
%*%
para Multiplicar Matrices en R -
Utilice el operador
%*%
para obtener el producto escalar de vectores en R - Conclusión
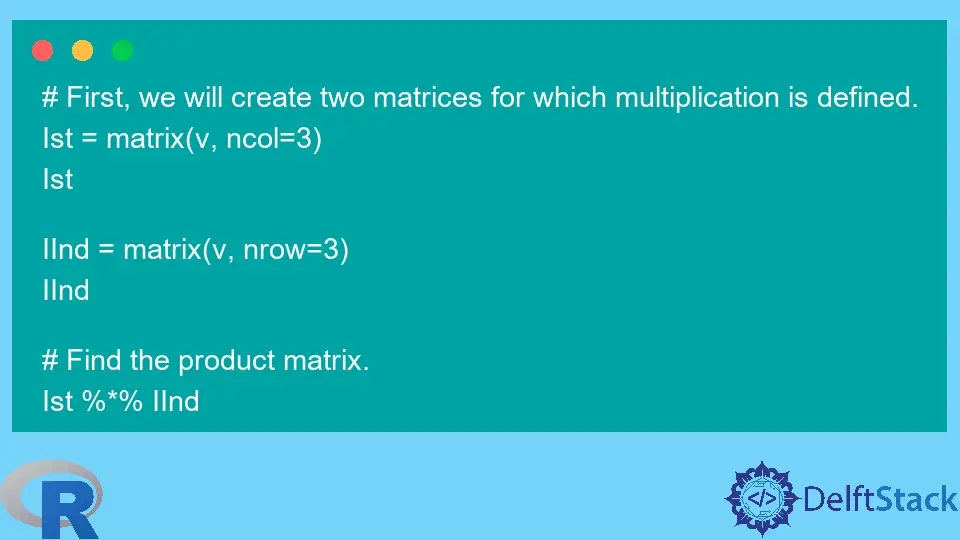
El operador %*%
se utiliza para la multiplicación de matrices. En vectores de la misma longitud, este operador da el producto escalar.
En este artículo, exploraremos el uso de este operador con algunos ejemplos simples.
Matrix y sus dimensiones en R
Una matriz es un arreglo rectangular de números. Es como una tabla de números, con filas y columnas.
El siguiente código crea y muestra cuatro matrices usando los mismos 12 números.
Código de ejemplo:
# First, we will create a vector of numbers.
# These 12 numbers are what we will place in our matrices.
v = 7:18
# Matrix with 2 rows and 6 columns.
matrix(v, nrow=2)
dim(matrix(v, nrow=2))
# Matrix with 3 rows and 4 columns.
matrix(v, nrow=3)
dim(matrix(v, nrow=3))
# Matrix with 4 rows and 3 columns.
matrix(v, nrow=4)
dim(matrix(v, nrow=4))
# Matrix with 6 rows and 2 columns.
matrix(v, nrow=6)
dim(matrix(v, nrow=6))
Producción :
> # Matrix with 2 rows and 6 columns.
> matrix(v, nrow=2)
[,1] [,2] [,3] [,4] [,5] [,6]
[1,] 7 9 11 13 15 17
[2,] 8 10 12 14 16 18
> dim(matrix(v, nrow=2))
[1] 2 6
> # Matrix with 3 rows and 4 columns.
> matrix(v, nrow=3)
[,1] [,2] [,3] [,4]
[1,] 7 10 13 16
[2,] 8 11 14 17
[3,] 9 12 15 18
> dim(matrix(v, nrow=3))
[1] 3 4
> # Matrix with 4 rows and 3 columns.
> matrix(v, nrow=4)
[,1] [,2] [,3]
[1,] 7 11 15
[2,] 8 12 16
[3,] 9 13 17
[4,] 10 14 18
> dim(matrix(v, nrow=4))
[1] 4 3
> # Matrix with 6 rows and 2 columns.
> matrix(v, nrow=6)
[,1] [,2]
[1,] 7 13
[2,] 8 14
[3,] 9 15
[4,] 10 16
[5,] 11 17
[6,] 12 18
> dim(matrix(v, nrow=6))
[1] 6 2
Cada matriz que creamos anteriormente tenía un número diferente de filas y columnas.
Una matriz se describe por el número de sus filas y columnas; esto se llama su dimensión. Una matriz con m
filas y n
columnas se denomina matriz m x n
, y se lee m por n.
Las matrices que creamos tenían las siguientes dimensiones: 2x6
, 3x4
, 4x3
y 6x2
.
Usar el Operador %*%
para Multiplicar Matrices en R
La multiplicación de matrices solo se define cuando el número de columna de la primera matriz es igual al número de filas de la segunda matriz. Cuando se cumple esta condición, podemos multiplicar esas dos matrices en ese orden usando el operador %*%
, y el producto también es una matriz.
La matriz de productos tendrá tantas filas como la primera matriz y tantas columnas como la segunda.
Código de ejemplo:
# First, we will create two matrices for which multiplication is defined.
Ist = matrix(v, ncol=3)
Ist
IInd = matrix(v, nrow=3)
IInd
# Find the product matrix.
Ist %*% IInd
Producción :
> # First, we will create two matrices for which multiplication is defined.
> Ist = matrix(v, ncol=3)
> Ist
[,1] [,2] [,3]
[1,] 7 11 15
[2,] 8 12 16
[3,] 9 13 17
[4,] 10 14 18
> IInd = matrix(v, nrow=3)
> IInd
[,1] [,2] [,3] [,4]
[1,] 7 10 13 16
[2,] 8 11 14 17
[3,] 9 12 15 18
> # Find the product matrix.
> Ist %*% IInd
[,1] [,2] [,3] [,4]
[1,] 272 371 470 569
[2,] 296 404 512 620
[3,] 320 437 554 671
[4,] 344 470 596 722
Veremos otro ejemplo de multiplicación de matrices válida y dos ejemplos donde la multiplicación de matrices no está definida.
Código de ejemplo:
# A 3 x 2 matrix.
IInd_b = matrix(20:25, nrow=3)
IInd_b
# A 2 x 6 matrix.
Ist_b = matrix(v, nrow=2)
Ist_b
# Matrix multiplication is defined between Ist and IInd_b.
Ist %*% IInd_b
# Multiplication is NOT defined in the following two cases.
IInd_b %*% Ist
Ist_b %*% IInd_b
Producción :
> # A 3 x 2 matrix.
> IInd_b = matrix(20:25, nrow=3)
> IInd_b
[,1] [,2]
[1,] 20 23
[2,] 21 24
[3,] 22 25
> # A 2 x 6 matrix.
> Ist_b = matrix(v, nrow=2)
> Ist_b
[,1] [,2] [,3] [,4] [,5] [,6]
[1,] 7 9 11 13 15 17
[2,] 8 10 12 14 16 18
> # Matrix multiplication is defined between Ist and IInd_b.
> Ist %*% IInd_b
[,1] [,2]
[1,] 701 800
[2,] 764 872
[3,] 827 944
[4,] 890 1016
> # Multiplication is NOT defined in the following two cases.
> IInd_b %*% Ist
Error in IInd_b %*% Ist : non-conformable arguments
> Ist_b %*% IInd_b
Error in Ist_b %*% IInd_b : non-conformable arguments
Utilice el operador %*%
para obtener el producto escalar de vectores en R
Los vectores se describen por su longitud y clase (y tipo).
Código de ejemplo:
# Create a vector.
vtr = c(11,22,33)
# Check that it is a vector.
is.vector(vtr)
# Length of the vector.
length(vtr)
# Class of the vector.
class(vtr)
# Type of the vector.
typeof(vtr)
Producción :
> # Create a vector.
> vtr = c(11,22,33)
> # Check that it is a vector.
> is.vector(vtr)
[1] TRUE
> # Length of the vector.
> length(vtr)
[1] 3
> # Class of the vector.
> class(vtr)
[1] "numeric"
> # Type of the vector.
> typeof(vtr)
[1] "double"
La longitud de un vector es el número de elementos (números) en él.
Cuando multiplicamos dos vectores de la misma longitud usando el operador %*%
, obtenemos el producto escalar de los vectores. R implícitamente trata el primer vector como una matriz de fila y el segundo vector como una matriz de columna y nos da la matriz del producto.
Devuelve una matriz 1x1
en lugar de un escalar. Podemos verificar esto usando las funciones is.vector()
y is.matrix()
.
En el siguiente código, primero obtendremos el producto escalar entre dos vectores de la misma longitud. Entonces obtendremos el mismo resultado usando matrices de dimensiones conformes.
Código de ejemplo:
# Four-element vectors.
V_I = 22:25
V_II = 2:5
# Dot product of vectors of the same dimension.
V_I %*% V_II
# Check the input and output.
is.vector(V_I)
is.matrix(V_I)
is.vector(V_I %*% V_II)
is.matrix(V_I %*% V_II)
# Create matrices of conformable dimensions (where matrix multiplication is defined).
m_I = matrix(V_I, nrow=1)
m_I
m_II = matrix(V_II, ncol=1)
m_II
# Matrix product.
m_I %*% m_II
Producción :
> # Four-element vectors.
> V_I = 22:25
> V_II = 2:5
> # Dot product of vectors of the same dimension.
> V_I %*% V_II
[,1]
[1,] 334
> # Check the input and output.
> is.vector(V_I)
[1] TRUE
> is.matrix(V_I)
[1] FALSE
> is.vector(V_I %*% V_II)
[1] FALSE
> is.matrix(V_I %*% V_II)
[1] TRUE
> # Create matrices of conformable dimensions (where matrix multiplication is defined).
> m_I = matrix(V_I, nrow=1)
> m_I
[,1] [,2] [,3] [,4]
[1,] 22 23 24 25
> m_II = matrix(V_II, ncol=1)
> m_II
[,1]
[1,] 2
[2,] 3
[3,] 4
[4,] 5
> # Matrix product.
> m_I %*% m_II
[,1]
[1,] 334
No podemos calcular el producto escalar si los vectores tienen diferentes longitudes.
Código de ejemplo:
# A three-element vector.
V_II_b = 6:8
# Dot product is not possible.
V_I %*% V_II_b
Producción :
> # A three-element vector.
> V_II_b = 6:8
> # Dot product is not possible.
> V_I %*% V_II_b
Error in V_I %*% V_II_b : non-conformable arguments
Conclusión
Para matrices conformes para la multiplicación, %*%
devuelve la matriz del producto. Para vectores de la misma longitud, devuelve el producto escalar como una matriz 1x1
.