Analizar HTML en PHP
-
Utilice
DomDocument()
para analizar HTML en PHP -
Use
simplehtmldom
para analizar HTML en PHP -
Use
DiDOM
para analizar HTML en PHP
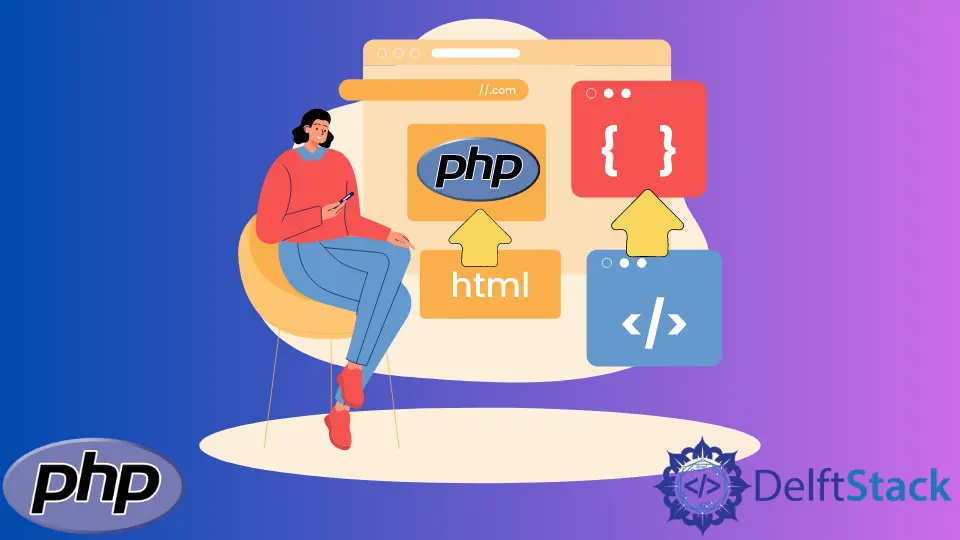
El análisis de HTML nos permite convertir su contenido o marcado en una cadena, lo que facilita el análisis o la creación de un archivo HTML dinámico. En más detalle, toma el código HTML sin procesar, lo lee, genera una estructura de objeto de árbol DOM desde los párrafos hasta los encabezados y nos permite extraer la información importante o necesaria.
Analizamos archivos HTML utilizando bibliotecas integradas y, a veces, bibliotecas de terceros para raspado web o análisis de contenido en PHP. Según el método, el objetivo es convertir el cuerpo del documento HTML en una cadena para extraer cada etiqueta HTML.
Este artículo discutirá la clase incorporada, DomDocument()
, y dos bibliotecas de terceros, simplehtmldom
y DiDOM
.
Utilice DomDocument()
para analizar HTML en PHP
Ya sea un archivo HTML local o una página web en línea, las clases DOMDocument()
y DOMXpath()
ayudan a analizar un archivo HTML y almacenar su elemento como cadenas o, en el caso de nuestro ejemplo, como un array.
Analicemos este archivo HTML usando las funciones y devolvamos los encabezados, subtítulos y párrafos.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<h2 class="main">Welcome to the Abode of PHP</h2>
<p class="special">
PHP has been the saving grace of the internet from its inception, it
runs over 70% of website on the internet
</p>
<h3>Understanding PHP</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
<h3>Using PHP</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
<h3>Install PHP</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
<h3>Configure PHP</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
<h2 class="main">Welcome to the Abode of JS</h2>
<p class="special">
PHP has been the saving grace of the internet from its inception, it
runs over 70% of website on the internet
</p>
<h3>Understanding JS</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
</body>
</html>
código PHP:
<?php
$html = 'index.html';
function getRootElement($element, $html)
{
$dom = new DomDocument();
$html = file_get_contents($html);
$dom->loadHTML($html);
$dom->preserveWhiteSpace = false;
$content = $dom->getElementsByTagName($element);
foreach ($content as $each) {
echo $each->nodeValue;
echo "\n";
}
}
echo "The H2 contents are:\n";
getRootElement("h2", $html);
echo "\n";
echo "The H3 contents are:\n";
getRootElement("h3", $html);
echo "\n";
echo "The Paragraph contents include\n";
getRootElement("p", $html);
echo "\n";
La salida del fragmento de código es:
The H2 contents are:
Welcome to the Abode of PHP
Welcome to the Abode of JS
The H3 contents are:
Understanding PHP
Using PHP
Install PHP
Configure PHP
Understanding JS
The Paragraph contents include
PHP has been the saving grace of the internet from its inception, it
runs over 70% of the website on the internet
...
Use simplehtmldom
para analizar HTML en PHP
Para funcionalidades adicionales como selectores de estilo CSS, puede usar una biblioteca de terceros llamada Simple HTML DOM Parser, que es un analizador de PHP simple y rápido. Puede descargarlo e incluir o requerir el archivo PHP único.
Con este proceso, puede analizar fácilmente todos los elementos que desee. Usando el mismo fragmento de código que en la sección anterior, analizaremos el HTML usando una función llamada str_get_html()
, que procesa el HTML y usa el método find()
para buscar un elemento o etiqueta HTML específico.
Para encontrar un elemento con una clase
especial, necesitamos que el selector de clase
se aplique a cada elemento find
. Además, para encontrar el texto real, necesitamos usar el selector innertext
en el elemento, que luego almacenamos en el array.
Usando el mismo archivo HTML que la última sección, analicémoslo usando el simplehtmldom
.
<?php
require_once('simple_html_dom.php');
function getByClass($element, $class)
{
$content= [];
$html = 'index.html';
$html_string = file_get_contents($html);
$html = str_get_html($html_string);
foreach ($html->find($element) as $element) {
if ($element->class === $class) {
array_push($heading, $element->innertext);
}
}
print_r($content);
}
getByClass("h2", "main");
getByClass("p", "special");
La salida del fragmento de código es:
Array
(
[0] => Welcome to the Abode of PHP
[1] => Welcome to the Abode of JS
)
Array
(
[0] => PHP has been the saving grace of the internet from its inception, it runs over 70% of the website on the internet
[1] => PHP has been the saving grace of the internet from its inception, it runs over 70% of the website on the internet
)
Use DiDOM
para analizar HTML en PHP
Para esta biblioteca de PHP de terceros, tenemos que usar un administrador de dependencias de PHP llamado Composer, que nos permite administrar todas nuestras bibliotecas y dependencias de PHP. La biblioteca DiDOM
está disponible a través de GitHub y proporciona más velocidad y administración de memoria que otras bibliotecas.
Si no lo tiene, puede instalar Composer aquí. Sin embargo, el siguiente comando agrega la biblioteca DiDOM
a su proyecto si la tiene.
composer require imangazaliev/didom
Después de eso, puedes usar el siguiente código, que tiene una estructura similar a simplehtmldom
con el método find()
. Hay un text()
, que convierte los contextos de los elementos HTML en cadenas que podemos usar en nuestro código.
La función has()
le permite verificar si tiene un elemento o una clase dentro de su cadena HTML y devuelve un valor booleano.
<?php
use DiDom\Document;
require_once('vendor/autoload.php');
$html = 'index.html';
$document = new Document('index.html', true);
echo "H3 Element\n";
if ($document->has('h3')) {
$elements = $document->find('h3');
foreach ($elements as $element) {
echo $element->text();
echo "\n";
}
}
echo "\nElement with the Class 'main'\n";
if ($document->has('.main')) {
$elements = $document->find('.main');
foreach ($elements as $element) {
echo $element->text();
echo "\n";
}
}
La salida del fragmento de código es:
H3 Element
Understanding PHP
Using PHP
Install PHP
Configure PHP
Understanding JS
Element with the Class 'main'
Welcome to the Abode of PHP
Welcome to the Abode of JS
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn