jQuery reproducir audio
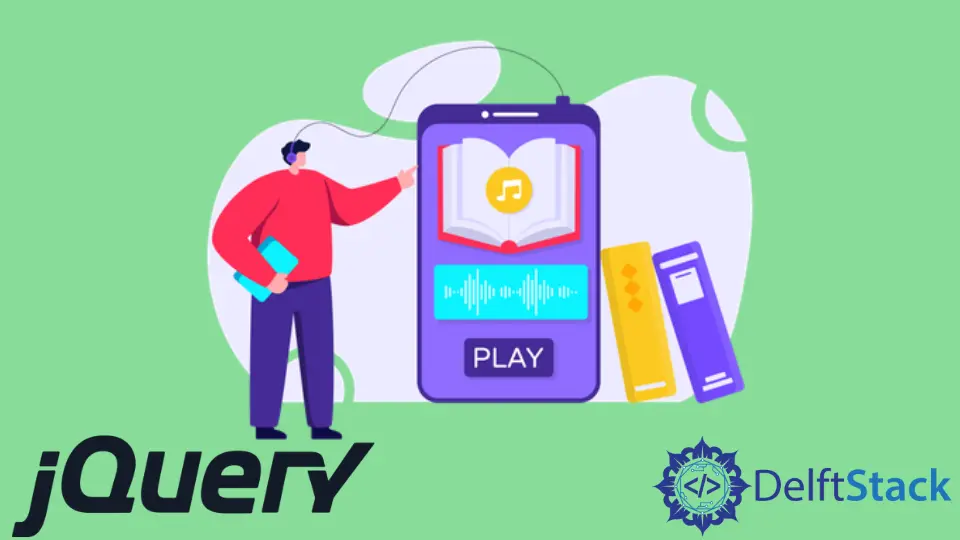
Este tutorial demuestra cómo reproducir audio usando jQuery.
Reproducir audio usando jQuery
jQuery no proporciona una funcionalidad integrada para reproducir audio, pero podemos usar la etiqueta HTML audio
con jQuery para crear un sistema para reproducir audio. Podemos manipular el evento canplay
de la etiqueta audio
para usarlo siempre que queramos reproducir audio usando jQuery.
Usando jQuery, podemos implementar opciones de reproducción, pausa, reinicio y parada para el audio. Creemos un sistema que pueda reproducir, pausar, reiniciar y detener el audio usando jQuery.
Vea los pasos a continuación:
-
Primero, la parte HTML solo contendrá divs y botones, no el elemento de audio.
-
jQuery creará el elemento de audio cuando el documento esté listo.
-
Una vez que se crea el elemento de audio, establecemos su atributo de origen.
-
Establezca el valor para el evento
terminado
. Lo hicimos reproducir nuevamente cuando el audio terminó de reproducirse. -
Implementamos tareas para los cuatro botones Reproducir, Pausa, Reiniciar y Detener.
-
Para los botones Reproducir y Pausa podemos usar los métodos integrados
reproducir()
ypausa()
. -
Para el reinicio, configuramos el
tiempo actual
para el audio en 0. Para la detención, configuramos eltiempo actual
para el audio en 0 y luego usamos el métodopausa ()
. -
Luego, usamos el evento
canplay
para mostrar la duración, la fuente y el estado del audio. -
Finalmente, mostramos el tiempo de reproducción del audio usando el evento
time update
.
Intentemos implementar un ejemplo basado en los pasos anteriores usando jQuery:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery Play Audio</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.2/themes/base/jquery-ui.css">
<style>
#Demo {
background-color : lightgreen;
height: 50%;
width: 100%;
}
#Main {
border: 5px solid green;
background-color : lightgreen;
height: 10%;
width: 20%;
}
.DemoButton {
font-size: 14px;
font-weight: 500;
line-height: 20px;
list-style: none;
cursor: pointer;
display: inline-block;
font-family: "Haas Grot Text R Web", "Helvetica Neue", Helvetica, Arial, sans-serif;
margin: 0;
padding: 10px 12px;
text-align: center;
background-color: rgba(51, 51, 51, 0.05);
border-radius: 8px;
border-width: 0;
color: #333333;
transition: all 200ms;
vertical-align: baseline;
white-space: nowrap;
user-select: none;
-webkit-user-select: none;
touch-action: manipulation;
}
</style>
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
</head>
<body>
<div id = "Main">
<h2>Click to Play, Pause, Restart, Stop...</h2>
<button class="DemoButton" id="ClickPlay">Play</button>
<button class="DemoButton" id="ClickPause">Pause</button>
<button class="DemoButton" id="ClickRestart">Restart</button>
<button class="DemoButton" id="ClickStop">Stop</button>
<hr>
<h2>Audio Playing Time</h2>
<div id="AudioRunningTime">0</div>
<hr>
<h2>Audio File Info</h2>
<div id="AudioLength">Duration of Audio:</div>
<div id="AudioSource">Source of the Audio:</div>
<div id="AudioStatus" style="color:red;">Status: Click Play</div>
</div>
</body>
<script>
$(document).ready(function() {
var DemoAudio = document.createElement('audio');
DemoAudio.setAttribute('src', 'demo.mp3');
DemoAudio.addEventListener('ended', function() {
this.play();
}, false);
DemoAudio.addEventListener("canplay",function(){
$("#AudioLength").text("Duration of Audio:" + DemoAudio.duration + " seconds");
$("#AudioSource").text("Source of the Audio:" + DemoAudio.src);
$("#AudioStatus").text("Status of Audio: Click Play").css("color","darkgreen");
});
DemoAudio.addEventListener("timeupdate",function(){
$("#AudioRunningTime").text("Current second:" + DemoAudio.currentTime);
});
$('#ClickPlay').click(function() {
DemoAudio.play();
$("#AudioStatus").text("Status: Playing the Audio");
});
$('#ClickPause').click(function() {
DemoAudio.pause();
$("#AudioStatus").text("Status: Paused the Audio");
});
$('#ClickRestart').click(function() {
DemoAudio.currentTime = 0;
});
$('#ClickStop').click(function() {
DemoAudio.currentTime = 0;
DemoAudio.pause();
$("#AudioStatus").text("Status: Stop the Audio");
});
});
</script>
</body>
</html>
El código anterior reproducirá el audio proporcionado en la fuente, y podemos reproducir, pausar, reiniciar y detener el audio en cualquier momento. Ver la salida:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook