Use If-Else y cambie la plantilla HTML de Loop Inside en Golang
-
Use el bucle
if-else
dentro de las plantillas HTML en Golang -
Utilice la plantilla HTML
switch
Loop Inside en Golang
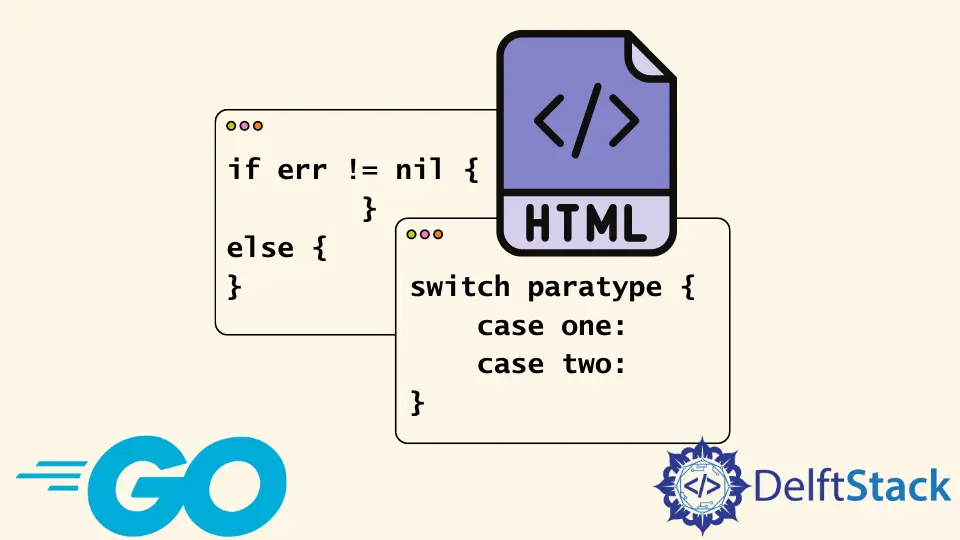
La plantilla HTML es un paquete Golang (Go) que admite plantillas basadas en datos para crear salidas HTML seguras contra la inyección de código. Una ventaja importante de utilizar HTML/plantilla es que genera una salida HTML segura y con escape mediante el escape automático contextual.
Como resultado, siempre que la salida sea HTML, siempre se debe usar el paquete de plantilla HTML en lugar de una plantilla de texto. Veamos algunos ejemplos de la utilización de if-else
y switch
en la plantilla HTML.
Use el bucle if-else
dentro de las plantillas HTML en Golang
En este ejemplo, creamos dos estructuras para almacenar los datos de los elementos de nuestra lista ToDo
: ToDo
y PageData
. La plantilla HTML definida, tmpl
, se crea y analiza.
Finalmente, renderizamos nuestro HTML dándolo a la función Execute()
, los datos y la plantilla HTML analizada.
Código:
package main
import (
"html/template"
"log"
"os"
)
type Todo struct {
Title string
Done bool
}
type PageData struct {
PageTitle string
Todos []Todo
}
func main() {
const tmpl = `
<h1>{{.PageTitle}}</h1>
<ul>
{{range .Todos}}
{{if .Done}}
<li>{{.Title}} ✔</li>
{{else}}
<li>{{.Title}}</li>
{{end}}
{{end}}
</ul>`
t, err := template.New("webpage").Parse(tmpl)
if err != nil {
log.Fatal(err)
}
data := PageData{
PageTitle: "Personal TODO list",
Todos: []Todo{
{Title: "Task 1", Done: true},
{Title: "Task 2", Done: false},
{Title: "Task 3", Done: false},
},
}
t.Execute(os.Stdout, data)
}
Producción :
Personal TODO list
Task 1 ✔
Task 2
Task 3
Utilice la plantilla HTML switch
Loop Inside en Golang
Código:
package main
import (
"fmt"
"html/template"
"os"
)
func main() {
const (
paragraph_hypothesis = 1 << iota
paragraph_attachment = 1 << iota
paragraph_menu = 1 << iota
)
const text = "{{.Paratype | printpara}}\n"
type Paragraph struct {
Paratype int
}
var paralist = []*Paragraph{
&Paragraph{paragraph_hypothesis},
&Paragraph{paragraph_attachment},
&Paragraph{paragraph_menu},
}
t := template.New("testparagraphs")
printPara := func(paratype int) string {
text := ""
switch paratype {
case paragraph_hypothesis:
text = "This is a hypothesis testing\n"
case paragraph_attachment:
text = "This is using switch case\n"
case paragraph_menu:
text = "Menu\n1:\n2:\n3:\n\nPick any option:\n"
}
return text
}
template.Must(t.Funcs(template.FuncMap{"printpara": printPara}).Parse(text))
for _, p := range paralist {
err := t.Execute(os.Stdout, p)
if err != nil {
fmt.Println("executing template:", err)
}
}
}
Producción :
This is a hypothesis testing
This is using switch case
Menu
1:
2:
3:
Pick any option: