Compruebe si un array contiene un valor en C#
-
Obtenga el índice de un elemento en un array con la función
Array.IndexOf()
enC#
-
Obtenga el índice de un elemento en un array con la función
Array.FindIndex()
enC#
-
Compruebe si hay un elemento en un array con
Array.Exists()
enC#
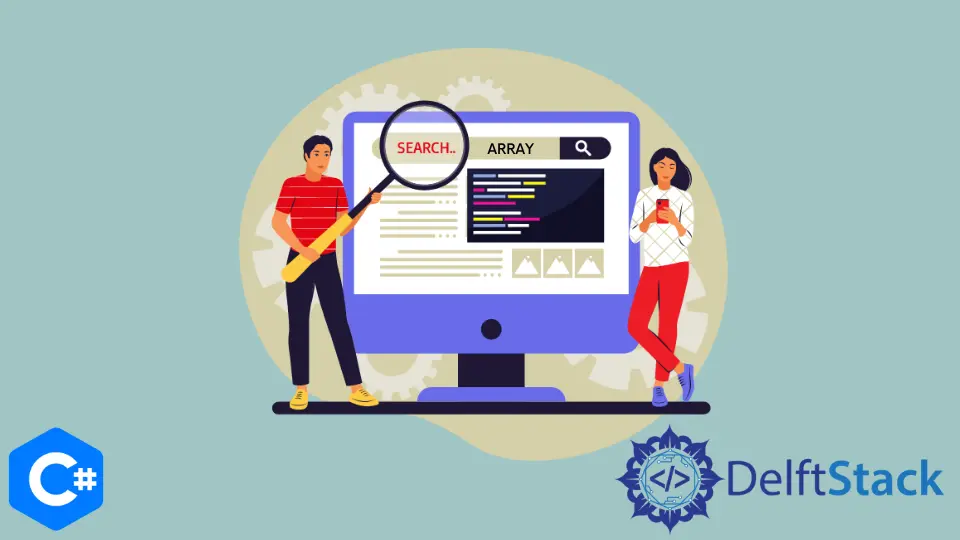
Este tutorial presentará métodos para verificar un elemento dentro de un array en C#.
Obtenga el índice de un elemento en un array con la función Array.IndexOf()
en C#
La función C# Array.IndexOf(array, element)
obtiene el índice del elemento elemento
dentro del array array
. Devuelve -1
si el elemento no está presente en el array.
El siguiente ejemplo de código nos muestra cómo podemos obtener el índice de un elemento en un array con la función Array.Indexof()
en C#.
using System;
namespace check_element_in_array {
class Program {
static void Main(string[] args) {
string[] stringArray = { "value1", "value2", "value3", "value4" };
string value = "value3";
int index = Array.IndexOf(stringArray, value);
if (index > -1) {
Console.WriteLine("{0} found in the array at index {1}", value, index);
} else {
Console.WriteLine("Value not found");
}
}
}
}
Producción :
value3 found in the array at index 2
Mostramos el índice del elemento value3
dentro del array stringArray
con la función Array.IndexOf()
en C#. El código anterior muestra el índice del elemento si se encuentra el valor y muestra valor no encontrado
si el valor no se encuentra en el array.
Obtenga el índice de un elemento en un array con la función Array.FindIndex()
en C#
La función Array.FindIndex(array, pattern)
obtiene el índice del elemento que coincide con el patrón pattern
dentro del array array
en C# si el elemento está presente en el array. Devuelve -1
si el elemento no está presente en el array. Podemos usar expresiones lambda para especificar el parámetro patrón
en la función Array.FindIndex()
.
El siguiente ejemplo de código nos muestra cómo podemos obtener el índice de un elemento en un array con la función Array.FindIndex()
y expresiones lambda en C#.
using System;
namespace check_element_in_array {
class Program {
static void Main(string[] args) {
string[] stringArray = { "value1", "value2", "value3", "value4" };
string value = "value3";
var index = Array.FindIndex(stringArray, x => x == value);
if (index > -1) {
Console.WriteLine("{0} found in the array at index {1}", value, index);
} else {
Console.WriteLine("Value not found");
}
}
}
}
Producción :
value3 found in the array at index 2
Mostramos el índice del elemento valor3
dentro del array stringArray
con la función Array.IndexOf()
en C#. El código anterior muestra el índice del elemento si se encuentra el valor y muestra valor no encontrado
si el valor no se encuentra en el array.
Compruebe si hay un elemento en un array con Array.Exists()
en C#
Si solo necesitamos verificar si un elemento existe en un array y no estamos preocupados por el índice del array donde se encuentra el elemento, podemos usar la función Array.Exists()
en C#. La función Array.Exists()
devuelve un valor booleano que es true
si el elemento existe en el array y false
si no existe en el array.
El siguiente ejemplo de código nos muestra cómo podemos verificar un elemento en un array con la función Array.Exists()
en C#.
using System;
namespace check_element_in_array {
class Program {
static void Main(string[] args) {
string[] stringArray = { "value1", "value2", "value3", "value4" };
string value = "value3";
var check = Array.Exists(stringArray, x => x == value);
if (check == true) {
Console.WriteLine("{0} found in the array", value);
} else {
Console.WriteLine("Value not found");
}
}
}
}
Producción :
value3 found in the array
En el código anterior, verificamos si el valor value3
existe en el array stringArray
con la función Array.Exists()
en C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn