Agregar un elemento a un ListBox en C# y WinForms
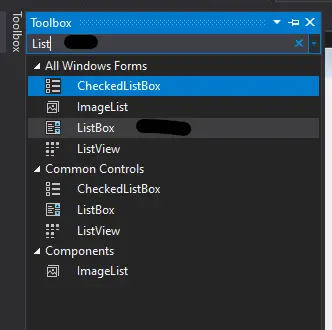
El control ListBox
se usa en WinForms para mostrar varios elementos en una lista, de la cual un usuario puede elegir uno o más elementos, y los componentes a menudo se presentan en varias columnas. Este tutorial discutirá cómo agregar un elemento a un ListBox
usando C# y WinForms.
Métodos para agregar un elemento a un ListBox
Método 1: arrastrar y soltar en WinForms
Para agregar elementos al ListBox
en WinForms, siga los pasos a continuación.
-
Cree un nuevo proyecto de
Windows Forms
enVisual Studio
y asígnele un nombre significativo -
Ahora, desde el panel izquierdo de
ToolBox
busqueListBox
-
Arrástrelo y suéltelo en el formulario de Windows
-
Para agregar nuevos elementos al
ListBox
, haga clic derecho en elListBox
-
En las Propiedades, busque
Elementos
y haga clic en los tres puntos, luego agregue elementos y haga clic enAceptar
-
Por último, ejecute el formulario de Windows
Producción:
Método 2: escribir código en C# para agregar elementos manualmente en ListBox
Puede agregar manualmente los elementos en el ListBox
escribiendo código. Veamos un ejemplo para comprender mejor este concepto.
-
Para comenzar, importe las siguientes bibliotecas
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms;
-
Cree una lista
ListBox
llamadashaniList
ListBox shaniList = new ListBox();
-
Luego, agregaremos el elemento a la lista como se muestra a continuación.
shaniList.Items.Add("Cut"); shaniList.Items.Add("Paste"); shaniList.Items.Add("Copy");
-
Agregue un control
ListBox
al formulario medianteControls.Add()
como último pasothis.Controls.Add(shaniList);
Código fuente completo:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace AddItemtoListBoxbyZeeshan {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {
ListBox shaniList = new ListBox();
shaniList.Location = new Point(150, 70);
shaniList.Items.Add("Cut");
shaniList.Items.Add("Paste");
shaniList.Items.Add("Copy");
this.Controls.Add(shaniList);
}
}
}
Producción:
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn