Elementos de vector inverso usando utilidades STL en C++
-
Utilice el algoritmo
std::reverse
para invertir elementos vectoriales en C++ -
Utilice el algoritmo
std::shuffle
para reordenar elementos vectoriales aleatoriamente en C++ -
Utilice el algoritmo
std::rotate
para rotar elementos vectoriales en C++
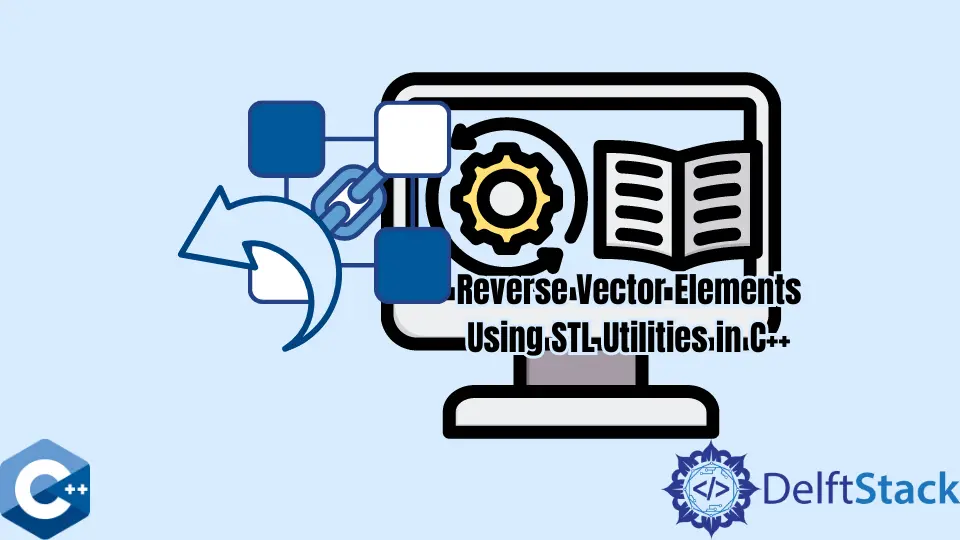
Este artículo presentará cómo revertir elementos vectoriales utilizando utilidades STL en C++.
Utilice el algoritmo std::reverse
para invertir elementos vectoriales en C++
std::reverse
es parte de los algoritmos STL y puede usarse para invertir el orden de los elementos en cualquier rango dado. El algoritmo std::reverse
intercambia internamente dos elementos comenzando por el primer y el último par. std::reverse
toma dos argumentos que representan iteradores del rango dado. Generamos enteros aleatorios como un objeto vector
en el siguiente ejemplo, que se invierte usando el algoritmo std::reverse
y envía los resultados al flujo cout
.
#include <iomanip>
#include <iostream>
#include <iterator>
#include <random>
#include <vector>
using std::cout;
using std::endl;
using std::left;
using std::setw;
void generateNumbers(std::vector<int> &arr, size_t &width) {
std::srand(std::time(nullptr));
for (size_t i = 0; i < width; i++) {
arr.push_back(std::rand() % 100);
}
}
int main() {
size_t width = 10;
std::vector<int> arr;
arr.reserve(width);
generateNumbers(arr, width);
cout << left << setw(10) << "arr: ";
copy(arr.begin(), arr.end(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
std::reverse(arr.begin(), arr.end());
cout << left << setw(10) << "arr: ";
copy(arr.begin(), arr.end(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
return EXIT_SUCCESS;
}
Producción :
arr: 78; 56; 63; 59; 16; 7; 54; 98; 87; 92;
arr: 92; 87; 98; 54; 7; 16; 59; 63; 56; 78;
Utilice el algoritmo std::shuffle
para reordenar elementos vectoriales aleatoriamente en C++
std::shuffle
se puede usar para reordenar aleatoriamente los elementos en el rango de modo que cada permutación de elementos tenga la misma probabilidad. La función toma al menos dos argumentos que denotan iteradores iniciales y finales del rango. Opcionalmente, std::shuffle
puede tomar el tercer argumento que representa la función generadora de números aleatorios. En este caso, utilizamos mersenne_twister_engine
, que se proporciona bajo el encabezado <random>
.
#include <iomanip>
#include <iostream>
#include <iterator>
#include <random>
#include <vector>
using std::cout;
using std::endl;
using std::left;
using std::setw;
void generateNumbers(std::vector<int> &arr, size_t &width) {
std::srand(std::time(nullptr));
for (size_t i = 0; i < width; i++) {
arr.push_back(std::rand() % 100);
}
}
int main() {
size_t width = 10;
std::vector<int> arr;
arr.reserve(width);
generateNumbers(arr, width);
cout << left << setw(10) << "arr: ";
copy(arr.begin(), arr.end(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
std::shuffle(arr.begin(), arr.end(), std::mt19937(std::random_device()()));
cout << left << setw(10) << "arr: ";
copy(arr.begin(), arr.end(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
return EXIT_SUCCESS;
}
Producción :
arr: 40; 77; 74; 41; 79; 21; 81; 98; 13; 90;
arr: 79; 41; 90; 77; 21; 81; 98; 74; 13; 40;
Utilice el algoritmo std::rotate
para rotar elementos vectoriales en C++
Otra función útil incluida en los algoritmos STL es - std::rotate
. La función desplaza elementos a la izquierda y envuelve los elementos que se mueven fuera del límite del vector. std::rotate
toma tres argumentos de tipo iteradores ForwardIt
y realiza la rotación para que el elemento señalado por el segundo argumento se mueva a la primera posición de la lista recién generada.
#include <iomanip>
#include <iostream>
#include <iterator>
#include <random>
#include <vector>
using std::cout;
using std::endl;
using std::left;
using std::setw;
void generateNumbers(std::vector<int> &arr, size_t &width) {
std::srand(std::time(nullptr));
for (size_t i = 0; i < width; i++) {
arr.push_back(std::rand() % 100);
}
}
int main() {
size_t width = 10;
std::vector<int> arr;
arr.reserve(width);
generateNumbers(arr, width);
cout << left << setw(10) << "arr: ";
copy(arr.begin(), arr.end(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
std::rotate(arr.begin(), arr.begin() + (width / 2), arr.begin() + width);
cout << left << setw(10) << "arr: ";
copy(arr.begin(), arr.end(), std::ostream_iterator<int>(cout, "; "));
cout << endl;
return EXIT_SUCCESS;
}
Producción :
arr: 75; 16; 79; 62; 53; 5; 77; 50; 31; 54;
arr: 5; 77; 50; 31; 54; 75; 16; 79; 62; 53;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook