How to Fill an Array in Scala
- Creating Array in Scala
-
Creating and Filling Array Using the
fill
Keyword in Scala - Creating and Filling Array Random Values in Scala
-
Creating and Filling Array Using the
list()
Elements in Scala -
Creating and Filling Array Using the
ofDim
Keyword in Scala
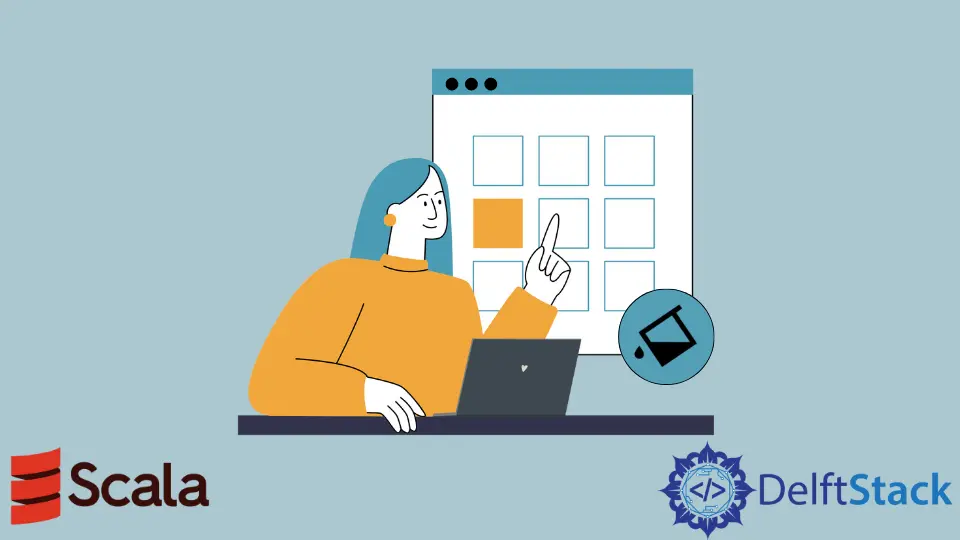
An array is a data structure and a container used to store similar data types. In Scala, the array is similar to Java and other programming languages.
Its index starts with 0
and ends at n-1
where n
is the array’s length. In this tutorial, we’ll talk about filling or initializing an array in Scala.
Creating Array in Scala
Use the Array
class and call its constructor by passing the values to create an array in Scala. Here, we created an arr
array that holds some integer values, and we used the foreach()
to traverse its elements.
object MyClass {
def main(args: Array[String]) {
val arr = Array(10,20,30)
arr.foreach(println)
}
}
Output:
10
20
30
Creating and Filling Array Using the fill
Keyword in Scala
The fill
keyword fills some initial or default values while creating the array instance. It requires some parameters such as type of values, array size, and the value to be filled.
In the following example, we used the foreach()
method to traverse the elements of the array.
object MyClass {
def main(args: Array[String]) {
val arr = Array.fill[Byte](5)(0)
arr.foreach(println)
}
}
Output:
0
0
0
0
0
Creating and Filling Array Random Values in Scala
Use the nextInt()
method to fill the array with random values. It generates the random integer values, and to get filled, we used the fill
keyword.
object MyClass {
def main(args: Array[String]) {
val arr = Array.fill(5){scala.util.Random.nextInt(5)}
arr.foreach(println)
}
}
Output:
4
3
4
2
1
Creating and Filling Array Using the list()
Elements in Scala
If you already have data into a list and want to convert that list into an array, use the below code example. The statement (list:_*)
copies all the list elements to the array during creating the instance.
object MyClass {
def main(args: Array[String]) {
val list = List(1,2,3,4,5)
val arr = Array[Int](list:_*)
arr.foreach(println)
}
}
Output:
1
2
3
4
5
Creating and Filling Array Using the ofDim
Keyword in Scala
The ofDim
keyword specifies the dimensions of an array during declaration. We will also specify the data type and size of the array in the next example.
The default value of int is 0
. So the array is initialized with 0
.
object MyClass {
def main(args: Array[String]) {
val arr = Array.ofDim[Int](5)
arr.foreach(println)
}
}
Output:
0
0
0
0
0