How to Set Checkbox Value in React
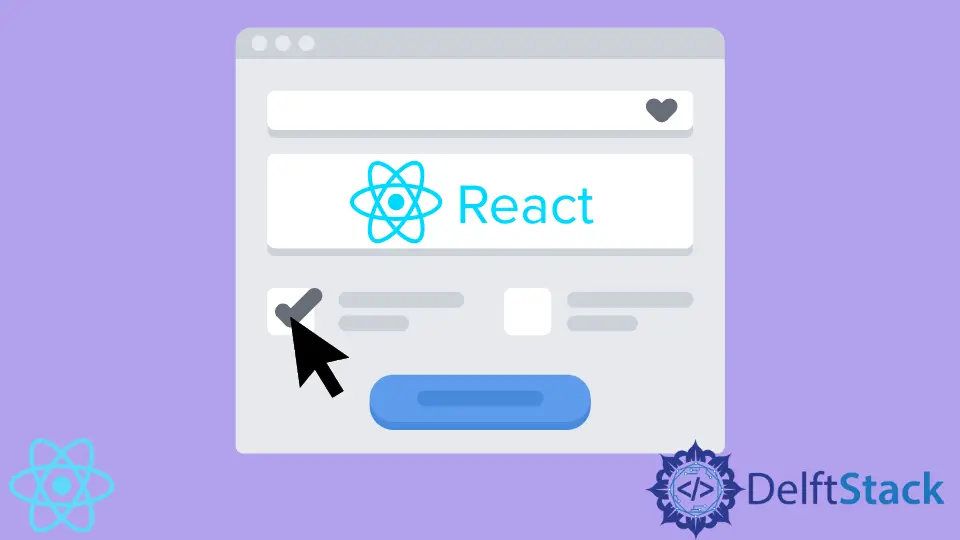
Every web application needs input elements where users can enter text, select a choice, or tick a checkbox. In this article, we will discuss how to handle input elements, specifically the checkboxes in React.
Checkbox With a Value-Controlled Input in React
Before we discuss implementing a controlled checkbox with a checked
value in React, let’s talk about controlled inputs.
In React, there are controlled and uncontrolled components. Inputs can be either one of these two types.
A component (or an input element) is controlled when it gets its value from the state, and the user’s actions update the state.
Here is what happens when users change the value in the input field:
- Set the field’s value from the
state
value. - Entering new characters into the field triggers an
onChange
event. - You set the handler to update the state to reflect the changes.
- New state value is displayed in the input field.
In other words, there is a two-way binding between the input element and the state. Changes in the input element update the state, and the element gets its value from the state.
Set the Checkbox Value in React
Typically, you can set an input element’s value
attribute to ensure it gets its value from the state. With checkboxes, we use the checked
attribute.
You can set the checked
attribute equal to the boolean value. Therefore, the state value needs to be true
or false
, not string, integer, or any other type.
Let’s look at this example:
export default function App() {
const [condition, setCondition] = useState(true);
return (
<div className="App">
Interested?
<input
type="checkbox"
checked={condition}
onChange={() => setCondition(!condition)}
/>
</div>
);
}
Check out the live demo on CodeSandbox.
In this example, we set the checked
attribute of the checkbox to the condition
state variable. We initialized the value of this state variable using the useState()
hook.
When initializing a state variable, the argument provided to the useState()
will be the initial value of the state variable. In this case, the condition
variable will be initialized as true
.
Next, update the state to reflect the user’s most recent input. For that, we set the onChange
attribute to update the state variable every time there’s a change to the component.
In this case, we set the condition
state variable to the opposite of its current value. If it’s currently true
, changing the checkbox (unticking it) will set it to false
, and if it’s currently false
(unticked), it will be set to true
.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn