How to Convert DateTime to String in PHP
-
Use
format()
Method inDateTime
Class -
Use
date_format()
Method -
Use Predefined Formats in
date_d.php
-
Use
list()
to ConvertDateTime
toString
in PHP
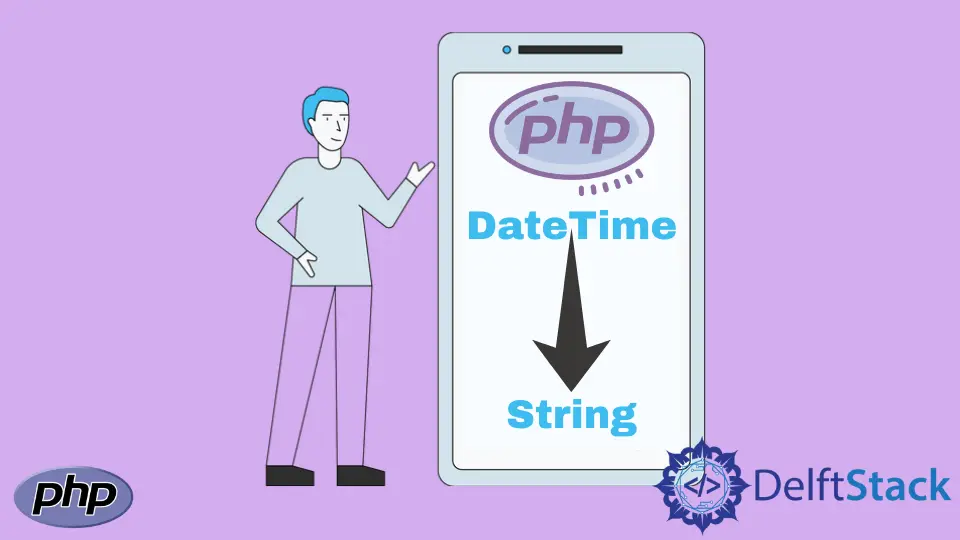
Converting DateTime
to string in PHP is quite easy, there are useful functions available that are meant for this purpose like format()
of DateTime
class, date_format()
, using the predefined formats or using list()
.
Use format()
Method in DateTime
Class
PHP has a class DateTime
for managing date and time. It’s been available since PHP version 5.2.
Some reasons why use DateTime
over traditional PHP functions to manage dates:
- It’s able to process more string formats
- It’s easier than other functions.
- The interface encapsulates back-end logic with a clean interface to use.
One of the useful methods of DateTime
class is the format()
, it returns formatted date string according to the given format.
Example:
$theDate = new DateTime('2020-03-08');
echo $stringDate = $theDate->format('Y-m-d H:i:s');
//output: 2020-03-08 00:00:00
The $stringDate
is a string with the provided format.
DateTime->format()
doesn’t really support microseconds.Use date_format()
Method
The date_format()
method will return a new DateTime
object and format it according to the given format.
Example:
$date = date_create_from_format('d M, Y', '08 Mar, 2020');
echo $newFormat = date_format($date,"Y/m/d H:i:s");
//output: 2020/03/08 00:00:00
According to the provided format, the date_create_from_format()
is being used to parses a time string. This function accepts three parameters: format
, time
, and the timezone
(optional).
In the example above, the date_format()
processes the created date to convert it to a string.
Use Predefined Formats in date_d.php
List of predefined formats:
define ('DATE_ATOM', "Y-m-d\TH:i:sP");
define ('DATE_COOKIE', "l, d-M-y H:i:s T");
define ('DATE_ISO8601', "Y-m-d\TH:i:sO");
define ('DATE_RFC822', "D, d M y H:i:s O");
define ('DATE_RFC850', "l, d-M-y H:i:s T");
define ('DATE_RFC1036', "D, d M y H:i:s O");
define ('DATE_RFC1123', "D, d M Y H:i:s O");
define ('DATE_RFC2822', "D, d M Y H:i:s O");
define ('DATE_RFC3339', "Y-m-d\TH:i:sP");
define ('DATE_RSS', "D, d M Y H:i:s O");
define ('DATE_W3C', "Y-m-d\TH:i:sP");
Example usage:
$dateFormat = new DateTime(); // this will return current date
echo $stringDate = $date->format(DATE_ATOM);
//output: 2020-03-08T12:54:56+01:00
The example above uses one of the predefined formats, so no need to actually provide it.
Use list()
to Convert DateTime
to String
in PHP
The list()
function can be used to assign values to a list of variables in one operation but only works with numerical arrays. When the array is assigned to multiple values, the first item in the array will be assigned to the first variable, and so on, till the end of the number of variables. Though the number of variables cannot be more than the length of the numerical array.
Example:
$date = explode("/",date('d/m/Y/h/i/s')
list($day,$month,$year,$hour,$min,$sec) = $date);
echo $month.'/'.$day.'/'.$year.' '.$hour.':'.$min.':'.$sec;
//output: 03/08/2020 02:01:06
Using the list()
function, you can easily use $day
, $month
, $year
, $hour
, $min
, and $sec
as a variable.
Related Article - PHP Date
- How to Add Days to Date in PHP
- How to Calculate the Difference Between Two Dates Using PHP
- How to Convert String to Date and Date-Time in PHP
- How to Convert One Date Format to Another in PHP
- How to Get the Current Date and Time in PHP
Related Article - PHP DateTime
- How to Get the Last Day of the Month With PHP Functions
- How to Calculate the Difference Between Two Dates Using PHP
- How to Get the Current Year in PHP
- How to Convert a Date to a Timestamp in PHP
- How to Convert a Timestamp to a Readable Date or Time in PHP