How to Deserialize JSON in Java
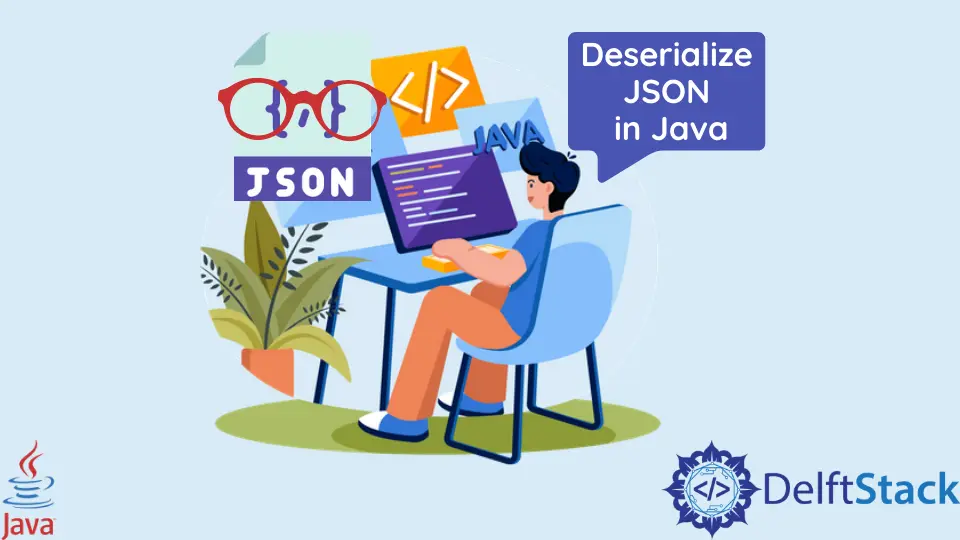
This tutorial demonstrates how to deserialize JSON in Java.
Deserialize JSON in Java
There are different libraries provided out there which are used for JSON operations. These libraries can also serialize and deserialize JSON objects in Java.
Deserialize JSON Using JSON-Java
API
There is a library JSON-Java
to handle the serialization and deserialization of JSON objects in Java. This library parses JSON objects or documents into Java objects or generates JSON objects documents from Java classes.
This library can be added using Gradle in Java. The Gradle dependency for JSON-Java
is:
implementation 'org.json:json:20210307'
The JSON-Java
comes with different features and is better than many other similar libraries. The main features are:
- Backward Compatibility
- There are no external dependencies.
- It has fast execution and a low memory footprint.
- Consistent and Reliable Results
- It provides adherence to the JSON specification.
import org.json.JSONObject;
public class Example {
public static void main(String[] args) {
String JSON_Object = "" "
{
"name" : "Sheeraz Gul",
"occupation" : "Software Engineer",
"salary" : "6000",
"height" : "186.6",
"married" : "false"
}
"" ";
var User_Info = new JSONObject(JSON_Object);
System.out.println(User_Info.get("name"));
System.out.println(User_Info.get("occupation"));
System.out.println(User_Info.get("salary"));
System.out.println(User_Info.get("height"));
System.out.println(User_Info.get("married"));
}
}
The code above will deserialize the JSON object into a Java object. See output:
Sheeraz Gul
Software Engineer
6000
186.6
false
Deserialize JSON Using Jackson
API
Jackson
is an open-source library for JSON operations in Java. The library can serialize and deserialize JSON objects in Java.
The library has two main methods for serialization and deserialization.
writeValue(...)
: Used to convert the Java object to JSON object. (Serialization)readValue(...)
: Used to convert JSON object to Java object. (Deserialization)
Jackson
can be used by adding it to the maven project. The dependency for Jackson
API is:
<dependency>
<groupId>org.codehaus.jackson</groupId>
<artifactId>jackson-mapper-asl</artifactId>
<version>1.9.13</version>
</dependency>
This dependency is for Jackson 1.x
; there is also a newer version, Jackson 2.x
. Let’s try an example to deserialize a JSON object to a Java object.
package delftstack;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.codehaus.jackson.JsonGenerationException;
import org.codehaus.jackson.map.JsonMappingException;
import org.codehaus.jackson.map.ObjectMapper;
public class Example {
public static void main(String[] args) {
ObjectMapper Object_Mapper = new ObjectMapper();
try {
// JSON string from file to java Object
User User_File = Object_Mapper.readValue(new File("G:\\delftstack.json"), User.class);
System.out.println(User_File);
// JSON string to java Object
String jsonInString = "{\"name\":Sheeraz,\"salary\":\"6000\", \"company\":\"Delftstack\"}";
User User_String = Object_Mapper.readValue(jsonInString, User.class);
System.out.println(User_String);
} catch (JsonGenerationException e) {
e.printStackTrace();
} catch (JsonMappingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
The code above will deserialize a JSON object to a Java object from a file and string. Both file and string contain the same JSON object.
See output:
User [name=Sheeraz, Salary=6000, Company=Delftstack]
User [name=Sheeraz, Salary=6000, Company=Delftstack]
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook