How to Get a Slice of Keys From a Map in Go
Jay Singh
Feb 02, 2024
-
Use
range
andappend()
to Get a Slice of Keys From a Map in Go -
Use the
MapKeys
Function to Get a Slice of Keys From a Map in Go
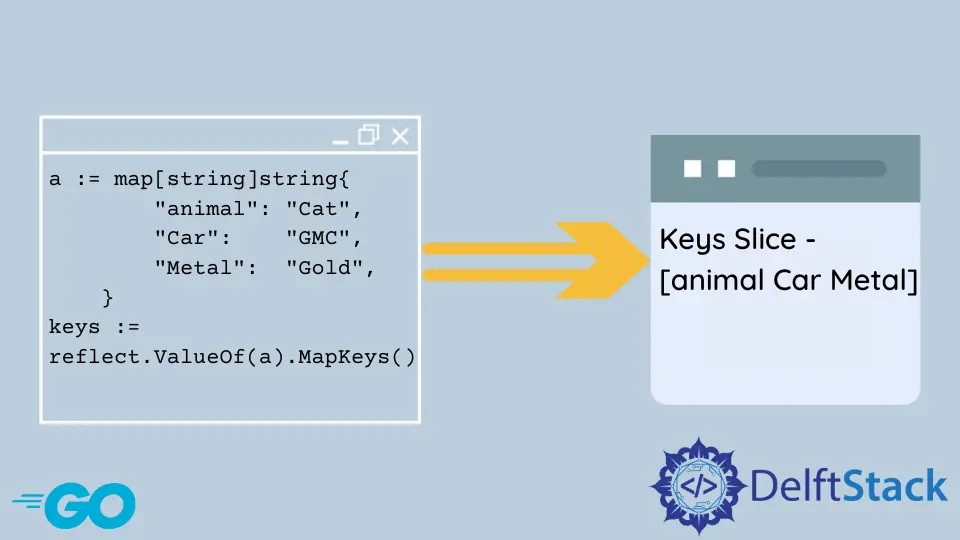
Golang Map is a collection of unordered key-value pairs. It is extensively used because it allows rapid lookups and values retrieved, updated, or deleted using keys.
Values in maps are not unique like keys and can be of any type, such as int, float64, rune, text, pointer, reference type, map type, etc.
In this tutorial, we’ll retrieve a slice of keys from a map in Go.
Use range
and append()
to Get a Slice of Keys From a Map in Go
We start with a map of string keys and string values in this example. Then we illustrate how to retrieve just the keys in a separate string slice.
We obtain the keys from the map by using range
and attaching them to an empty slice using append()
.
package main
import "fmt"
func main() {
m := map[string]string{
"animal": "Cat",
"Car": "GMC",
"Metal": "Gold",
}
keys := []string{}
for key, _ := range m {
keys = append(keys, key)
}
fmt.Println("KEYS SLICE -", keys)
}
Output:
KEYS SLICE - [animal Car Metal]
Use the MapKeys
Function to Get a Slice of Keys From a Map in Go
To acquire an array of keys of type []Value
, we use the MapKeys
method from the reflect package.
package main
import (
"fmt"
"reflect"
)
func main() {
a := map[string]string{
"animal": "Cat",
"Car": "GMC",
"Metal": "Gold",
}
keys := reflect.ValueOf(a).MapKeys()
fmt.Println("Keys Slice -", keys)
}
Output:
Keys Slice - [animal Car Metal]