How to Reverse a String in C#
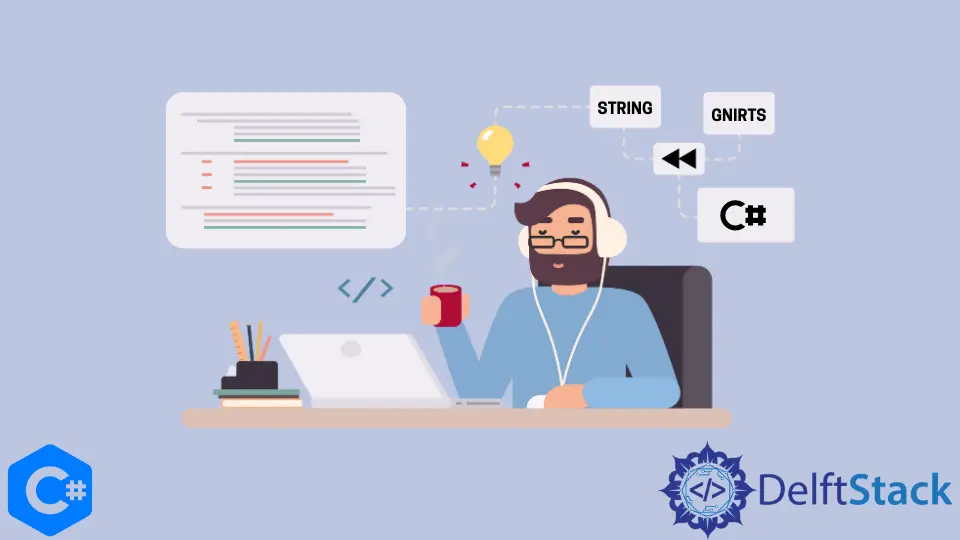
This tutorial will introduce methods to reverse the contents of a string variable in C#.
Reverse a String With the for
Loop in C#
The for
loop iterates through a specific section of code for a fixed amount of times in C#. We can use a for
loop to reverse the contents of a string variable. See the below example code.
using System;
namespace reverse_string {
class Program {
static string Reverse(string text) {
char[] charArray = text.ToCharArray();
string reverse = String.Empty;
for (int i = charArray.Length - 1; i >= 0; i--) {
reverse += charArray[i];
}
return reverse;
}
static void Main(string[] args) {
string original = "This is original";
string reversed = Reverse(original);
Console.WriteLine(reversed);
}
}
}
Output:
lanigiro si sihT
In the above code, we converted the string to the array of characters charArray
with the ToCharArray()
function. We then reversed the contents of the charArray
and sequentially concatenated the elements in the string variable reverse
. In the end, we returned the reverse
variable and printed it.
Reverse a String With the Array.Reverse()
Method in C#
The Array.Reverse(arr)
method reverses the sequence of elements inside the arr
array in C#. We reverse a string by converting it into an array of characters and reversing the character array with the Array.Reverse()
function. The following code example shows us how we can reverse a string with the Array.Reverse()
function in C#.
using System;
namespace reverse_string {
class Program {
static string Reverse(string text) {
char[] charArray = text.ToCharArray();
Array.Reverse(charArray);
return new string(charArray);
}
static void Main(string[] args) {
string original = "This is original";
string reversed = Reverse(original);
Console.WriteLine(reversed);
}
}
}
Output:
lanigiro si sihT
We first converted the string variable text
to the character array charArray
. We then reversed the contents inside the charArray
with the Array.Reverse(charArray)
function. We cast the charArray
to a string and returned the value. We stored the returned value into the string variable reversed
and printed it.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn