Float vs Double vs Decimal in C#
-
The Float data type in
C#
-
The Double data type in
C#
-
The Decimal data type in
C#
-
Float vs Double vs Decimal in
C#
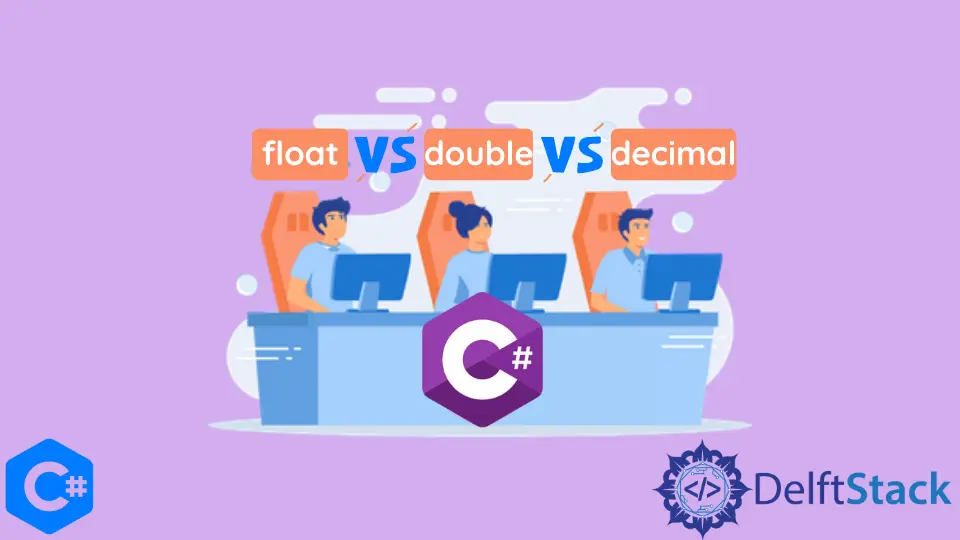
This tutorial will discuss the similarities and differences between Float, Double, and Decimal data types in C#.
The Float data type in C#
The Float data type stores floating-point values in C#. The float
keyword is used to declare a float variable. We have to use the f
specifier during initialization to tell the compiler that the value is a floating-point value. A float variable is 32bits
in size meaning that it can store values from -3.402823e38
to +3.402823e38
. A float variable has a precision of 7
digits in C#. The following code example shows us how we can declare a float variable in C#.
using System;
namespace data_types {
static void Main(string[] args) {
float fval = 100.0f / 3;
Console.WriteLine(fval);
}
}
}
Output:
33.33333
We initialized the float variable fval
with the float
keyword and the f
specifier in C#. The printed output shows that the precision of the fval
is only 7
digits.
The Double data type in C#
The Double data type also stores floating-point values in C#. The double
keyword is used to declare a double variable. We have to use the d
specifier during initialization to tell the compiler that the value is a double value. A double variable is 64bits
in size meaning that it can store values from -1.79769313486232e308
to +1.79769313486232e308
. A double variable has a precision of 15
digits in C#. The following code example shows us how we can declare a double variable in C#.
using System;
namespace data_types {
static void Main(string[] args) {
double dval = 100.0d / 3;
Console.WriteLine(dval);
}
}
}
Output:
33.3333333333333
We initialized the double variable dval
with the double
keyword and the d
specifier in C#. The printed output shows that the precision of the dval
is 15
digits.
The Decimal data type in C#
The Decimal data type also stores floating-point values in C#. The decimal
keyword is used to declare a decimal variable. We have to use the M
specifier during initialization to tell the compiler that the value is a decimal value. A decimal variable is 128bits
in size meaning that it can store values from -79,228,162,514,264,337,593,543,950,335
to +79,228,162,514,264,337,593,543,950,335
. A decimal variable has a precision of 29
digits in C#. The following code example shows us how we can declare a decimal variable in C#.
using System;
namespace data_types {
static void Main(string[] args) {
decimal dval = 100.0M / 3;
Console.WriteLine(dval);
}
}
}
Output:
33.333333333333333333333333333
We initialized the decimal variable dval
with the decimal
keyword and the M
specifier in C#. The printed output shows that the precision of the dval
is 29
digits.
Float vs Double vs Decimal in C#
The float
and double
data types are lower in precision and size than the decimal
data type. So, the decimal
data type is recommended for recording sensitive information that requires a large number of significant figures like financial transactions. But, the decimal
data type is much slower than the float
and double
data types. So, whenever we are dealing with some scientific values that are already an approximation and do not require very high precision, we can either use the float
or the double
data type depending upon our needs.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp Float
- How to Convert a String to Float in C#
- How to Convert Int to Float in C#
- How to Convert Float to Int in C#
- How to Generate a Random Float in C#