How to Properly Exit an Application in C#
-
Exit a Console Application With the
Environment.Exit()
Function inC#
-
Exit a Console Application With the
Application.Exit()
Function inC#
-
Properly Exit an Application With the
Environment.Exit()
andApplication.Exit()
Functions inC#
-
Exit the Application with the
Application.ExitThread()
Method - Conclusion
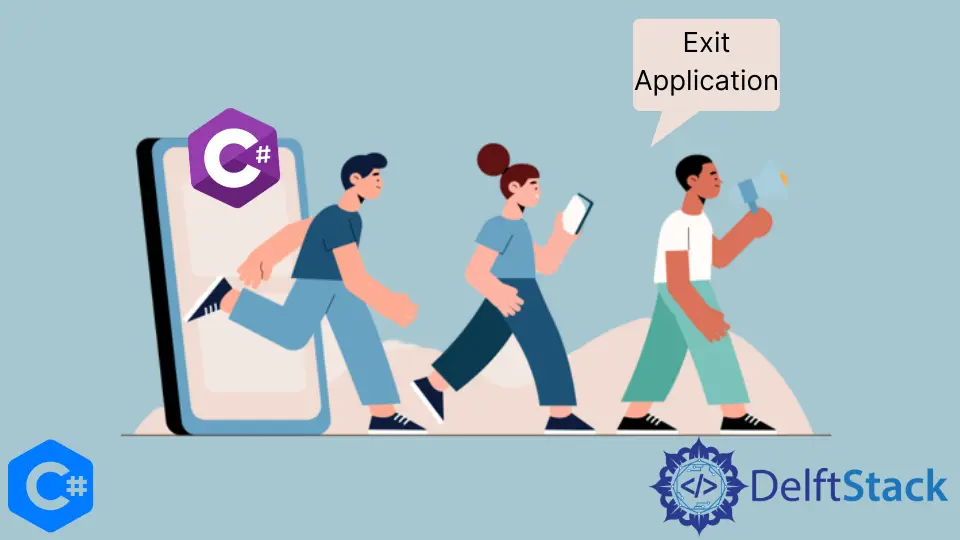
Exiting a C# application gracefully is a critical aspect of software development, and C# offers several methods to achieve this goal. Whether you want to terminate the entire application or exit specific threads while preserving others, understanding these methods is essential for ensuring clean and controlled application termination.
In this article, we will explore different methods for exiting a C# application, including Environment.Exit()
, Application.Exit()
, and Application.ExitThread()
. We will delve into their use cases, scenarios, and best practices.
Exit a Console Application With the Environment.Exit()
Function in C#
The Environment.Exit(exitCode)
function is used to terminate an entire application with the exitCode
as the exit code in C#. The Environment.Exit()
function terminates the entire current application and returns an exit code to the current operating system.
The Environment.Exit()
method is part of the System
namespace in C# and provides a means to forcefully terminate an application.
It’s a powerful tool for ensuring that an application can exit cleanly, even in the presence of unexpected errors or exceptional conditions. Here’s a breakdown of how this method works:
Method Signature
The Environment.Exit()
method has a single parameter: an integer representing the exit code. The exit code is a numeric value that communicates the reason for the application’s termination to the operating system.
By convention, a value of 0
typically indicates a successful and normal exit, while non-zero values can be used to indicate errors or specific exit conditions.
Basic Usage
To use the Environment.Exit()
method, you simply call it within your C# code, passing an appropriate exit code as an argument. Here’s a basic example:
Environment.Exit(0); // Exit the application with a success code
In this example, the application is exited with an exit code of 0
, indicating a successful termination.
Normal Application Exit
See the below example code.
using System;
namespace exit_application {
class Program {
static void Main(string[] args) {
Console.WriteLine("The Text before Exit");
Environment.Exit(0);
Console.WriteLine("The Text after Exit");
}
}
}
Output:
The Text before Exit
The above code only prints The Text before Exit
because we exit the application completely with the Environment.Exit(0)
function before the line Console.WriteLine("The Text after Exit");
. The Environment.Exit()
function can be used with both console-based applications and WinForms applications.
Handling an Error Exit
Let’s say you’re building a command-line utility, and an error occurs during its execution. You can use Environment.Exit()
to exit the application with an appropriate error code:
using System;
class Program {
static void Main() {
try {
// Simulate an error
throw new Exception("An error occurred.");
} catch (Exception ex) {
Console.WriteLine($"Error: {ex.Message}");
// Exit the application with a non-zero exit code to indicate an error
Environment.Exit(1);
}
}
}
Output:
Error: An error occurred.
Error: Command failed: timeout 7 mono HelloWorld.exe
In this example, when an error is encountered, the application exits with an exit code of 1
, indicating that an error occurred.
Exit a Console Application With the Application.Exit()
Function in C#
The Application.Exit()
function terminates all the message loops started with the Application.Run()
function and then exists all the windows of the current application in C#.
The Application.Exit()
method is a part of the System.Windows.Forms namespace and is primarily used in Windows Forms applications to facilitate a controlled and graceful exit.
Unlike some other methods that forcefully terminate an application, Application.Exit()
allows your application to perform necessary cleanup operations and handle events before it exits. Let’s delve into the details of how this method works and when to use it.
Method Signature
The Application.Exit()
method has a simple signature, requiring no parameters. You can call it directly in your C# code to initiate the exit process:
System.Windows.Forms.Application.Exit();
Using the Application.Exit()
method is straightforward. You typically place the call to this method in a handler for a user action or an application event that indicates the need to exit. Here’s a basic example:
using System;
using System.Windows.Forms;
namespace MyWindowsApp {
public partial class MainForm : Form {
public MainForm() {
InitializeComponent();
}
private void exitButton_Click(object sender, EventArgs e) {
// Perform any necessary cleanup or validation here
// ...
// Exit the application gracefully
System.Windows.Forms.Application.Exit();
}
}
}
In this example, the exitButton_Click
event handler is triggered when a button is clicked. Before calling Application.Exit()
, you can add code to perform any necessary cleanup or validation specific to your application.
We closed a WinForms application and all the threads associated with it with the Application.Exit()
function in C#. This method is preferable to the Environment.Exit()
function because the Environment.Exit()
function does not terminate all the application’s message loops.
Properly Exit an Application With the Environment.Exit()
and Application.Exit()
Functions in C#
We can use the combination of the Environment.Exit()
and Application.Exit()
functions to properly exit an application in C#. The following code example shows us how we can adequately close an application with a combination of the Environment.Exit()
and Application.Exit()
functions in C#.
using System;
using System.Windows.Forms;
if (Application.MessageLoop == true) {
Application.Exit();
} else {
Environment.Exit(1);
}
In the above code, we close the application with the Application.Exit()
function if the Application.Run()
function has been called before in the application. Otherwise, we close the application with the Environment.Exit(1)
function by giving 1
as the exit code to the operating system.
Exit the Application with the Application.ExitThread()
Method
The Application.ExitThread()
method is part of the System.Windows.Forms namespace and is typically used in Windows Forms applications.
Unlike some other methods that exit the entire application, Application.ExitThread()
enables you to exit a specific application thread, leaving other threads running and preserving the application’s functionality.
Method Signature
The Application.ExitThread()
method requires a single parameter, which is an instance of the Thread
class representing the thread to exit. It allows you to specify precisely which thread to terminate:
System.Windows.Forms.Application.ExitThread(Thread thread);
Using the Application.ExitThread()
method is straightforward. You typically place the call to this method in a handler for a user action or an event that signals the need to exit a specific thread. Here’s a basic example:
using System;
using System.Threading;
using System.Windows.Forms;
namespace MyWindowsApp {
public partial class MainForm : Form {
private Thread workerThread;
public MainForm() {
InitializeComponent();
}
private void startButton_Click(object sender, EventArgs e) {
// Create and start a new thread
workerThread = new Thread(WorkerMethod);
workerThread.Start();
}
private void stopButton_Click(object sender, EventArgs e) {
// Check if the workerThread is running
if (workerThread != null && workerThread.IsAlive) {
// Exit the workerThread gracefully
System.Windows.Forms.Application.ExitThread(workerThread);
}
}
private void WorkerMethod() {
// Simulate work in the worker thread
Thread.Sleep(5000);
}
}
}
In this example, the application starts a worker thread when the Start
button is clicked. The Stop
button triggers the stopButton_Click
event handler, which checks if the worker thread is running and, if so, gracefully exits it using Application.ExitThread(workerThread)
.
Conclusion
In this comprehensive article, we’ve explored various methods for exiting a C# application, each serving distinct purposes and offering precise control over the termination process.
Environment.Exit()
: This method allows you to terminate the entire application with an exit code, making it suitable for signaling success or handling errors. It’s a versatile tool for ensuring clean exits, even in the presence of unexpected errors or exceptional conditions.Application.Exit()
: Primarily used in Windows Forms applications, this method facilitates controlled and graceful exits. It allows for cleanup operations and event handling before exiting, ensuring a user-friendly experience and resource management.Application.ExitThread()
: In multi-threaded applications, this method enables you to exit specific threads while keeping others alive. It preserves the application’s functionality and prevents resource leaks, offering fine-grained control over thread termination.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn