How to Popup A Message in C#
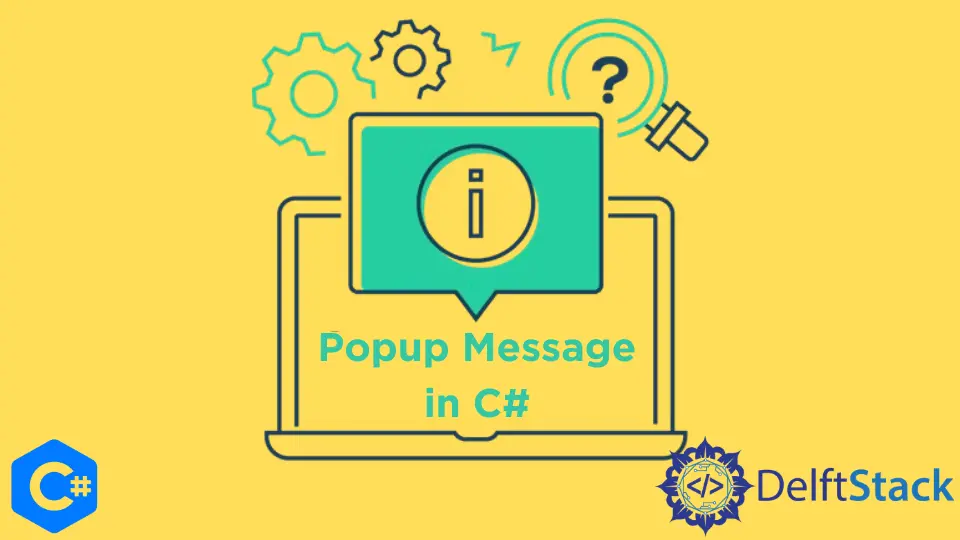
This tutorial will discuss the method of displaying a popup message window in C#.
Display Popup Message With the MessageBox
Class in C#
If we have a button and want to show a popup message when the button is clicked, we can use the MessageBox
class in C#. The MessageBox.Show()
method displays a message window on-screen in C#. The MessageBox.Show()
method takes the message in the string format as an input parameter and displays it to the user. The following code example shows us how to create a simple popup message window that shows some message in response to a button click with the MessageBox.Show()
method in C#.
using System;
using System.Windows.Forms;
namespace popup {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
string text = "This is some Text that I wanted to show";
MessageBox.Show(text);
}
}
}
Output:
In the above code, we created a popup message window that gets shown each time the button1
is clicked by the user with the MessageBox.Show()
function in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn