How to Check Number of Arguments in Linux Bash
- Understanding Bash Arguments
- Using Conditional Statements
- Looping Through Arguments
- Handling Optional Arguments
- Conclusion
- FAQ
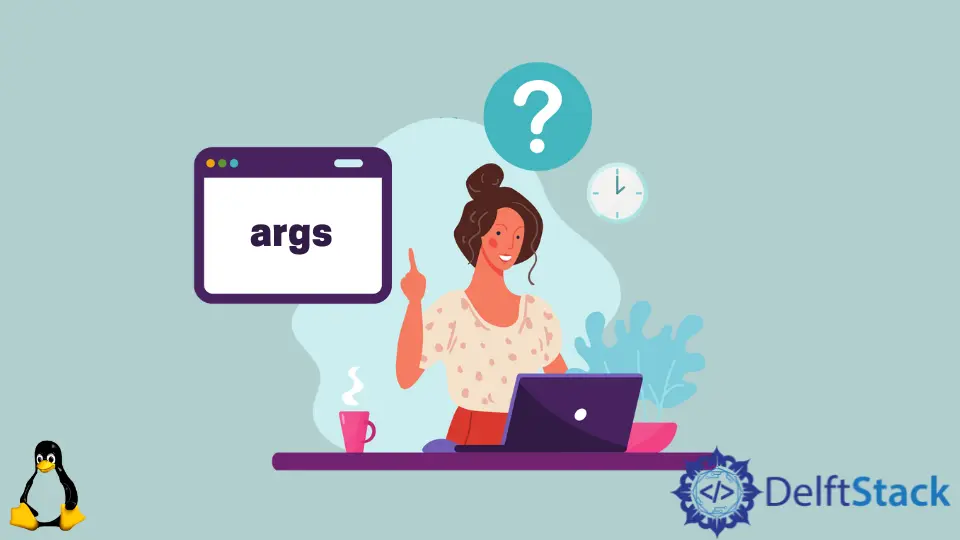
In the world of Linux Bash scripting, understanding how to check the number of arguments passed to a script is essential for creating robust and user-friendly scripts. Whether you’re writing a simple script or a complex automation tool, knowing how to handle arguments can make a significant difference in your script’s functionality.
This article will guide you through the different methods to check the number of arguments in Linux Bash, ensuring you can effectively manage input and enhance your scripts. From basic techniques to more advanced practices, we’ll cover it all in a conversational and easy-to-follow manner.
Understanding Bash Arguments
In Bash, when you run a script, you can pass additional information called arguments. These arguments can be accessed within the script using special variables. The first argument is referred to as $1
, the second as $2
, and so on. To check the total number of arguments provided, you can use the special variable $#
. This variable holds the count of all the arguments passed to the script.
Example of Checking Arguments
Here’s a straightforward example to illustrate how to check the number of arguments in a Bash script.
#!/bin/bash
echo "Number of arguments: $#"
When you run this script with a few arguments, it will output the total count.
Output:
Number of arguments: 3
In this example, if you execute the script with three arguments, the output will reflect that count. This technique is fundamental and serves as the basis for more complex argument handling in scripts.
Using Conditional Statements
Another effective way to manage arguments is through conditional statements. This allows you to perform different actions based on the number of arguments provided. For instance, you might want to check if the required number of arguments is present before proceeding.
Example of Conditional Argument Check
Here’s a script that checks if the user has provided the correct number of arguments.
#!/bin/bash
if [ $# -ne 2 ]; then
echo "Error: You must provide exactly 2 arguments."
exit 1
fi
echo "You provided the correct number of arguments."
When you run this script without the required two arguments, it will display an error message.
Output:
Error: You must provide exactly 2 arguments.
In this script, the conditional statement checks if the number of arguments ($#
) is not equal to 2. If the condition is true, it prints an error message and exits the script. This method is particularly useful for ensuring that your scripts receive the necessary input to function correctly.
Looping Through Arguments
Sometimes, you may want to process each argument individually. Looping through the arguments allows you to perform actions on each one, making your script more dynamic and flexible.
Example of Looping Through Arguments
Here’s an example of how to loop through all the arguments passed to a script.
#!/bin/bash
for arg in "$@"; do
echo "Argument: $arg"
done
When you run this script with multiple arguments, it will display each one.
Output:
Argument: first
Argument: second
Argument: third
In this script, the for
loop iterates over all the arguments provided to the script, represented by "$@"
. This method is useful for scenarios where you need to process or validate each argument separately, enhancing the script’s versatility.
Handling Optional Arguments
In some cases, you might want to make certain arguments optional while still enforcing requirements on others. This can be accomplished using a combination of conditional statements and argument checks.
Example of Optional Arguments
Here’s a script that demonstrates how to handle optional arguments.
#!/bin/bash
if [ $# -lt 1 ]; then
echo "Error: At least one argument is required."
exit 1
fi
echo "You provided at least one argument."
if [ $# -eq 2 ]; then
echo "You provided exactly 2 arguments."
fi
When you run this script with one or two arguments, it will respond accordingly.
Output:
You provided at least one argument.
This script first checks if at least one argument is provided. If not, it shows an error message. Then, it checks if exactly two arguments were supplied, demonstrating how to manage both required and optional inputs effectively.
Conclusion
Mastering how to check the number of arguments in Linux Bash is crucial for anyone looking to write effective scripts. Whether you’re using simple checks, conditional statements, or loops, understanding these concepts will greatly enhance your scripting capabilities. By implementing the techniques discussed in this article, you can create scripts that are not only functional but also user-friendly. With practice, you’ll be able to handle arguments with ease, making your scripts robust and efficient.
FAQ
- How can I check if no arguments are passed to my Bash script?
You can check if no arguments are passed by usingif [ $# -eq 0 ]; then
.
-
Is it possible to pass more than one argument to a Bash script?
Yes, you can pass multiple arguments separated by spaces when executing the script. -
What does the special variable
$@
do in Bash?
The variable$@
represents all the arguments passed to the script as separate words. -
Can I use default values for missing arguments in Bash?
Yes, you can set default values using conditional checks or by assigning values if an argument is not provided. -
How do I access the first argument in a Bash script?
You can access the first argument using$1
.
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn